c++定义时间(CMyTime),有小时、分钟和秒三个成员变量,重载 “-” (减)、后置“++”和“>=”运算符,实现函数 show 显示时间,测试 MyTime。
时间: 2024-03-14 14:42:55 浏览: 23
好的,我来为您解答。
首先,可以这样定义 CMyTime 类:
```cpp
class CMyTime {
public:
CMyTime(int h = 0, int m = 0, int s = 0) : hour(h), minute(m), second(s) {}
CMyTime operator-(const CMyTime& t) const;
CMyTime operator++(int);
bool operator>=(const CMyTime& t) const;
void show() const;
private:
int hour;
int minute;
int second;
};
```
然后,实现这几个运算符的重载:
```cpp
CMyTime CMyTime::operator-(const CMyTime& t) const {
int s = second - t.second;
int m = minute - t.minute;
int h = hour - t.hour;
if (s < 0) {
s += 60;
--m;
}
if (m < 0) {
m += 60;
--h;
}
return CMyTime(h, m, s);
}
CMyTime CMyTime::operator++(int) {
CMyTime t(*this);
second++;
if (second >= 60) {
second -= 60;
minute++;
if (minute >= 60) {
minute -= 60;
hour++;
if (hour >= 24) {
hour = 0;
}
}
}
return t;
}
bool CMyTime::operator>=(const CMyTime& t) const {
if (hour > t.hour || (hour == t.hour && minute > t.minute) || (hour == t.hour && minute == t.minute && second >= t.second)) {
return true;
}
else {
return false;
}
}
void CMyTime::show() const {
cout << setfill('0') << setw(2) << hour << ":" << setw(2) << minute << ":" << setw(2) << second << endl;
}
```
最后,可以在 main 函数中测试 CMyTime:
```cpp
int main() {
CMyTime t1(10, 20, 30);
CMyTime t2(2, 45, 10);
CMyTime t3 = t1 - t2;
t3.show();
t1++;
t1.show();
if (t1 >= t2) {
cout << "t1 is later than or equal to t2" << endl;
}
else {
cout << "t1 is earlier than t2" << endl;
}
return 0;
}
```
输出结果如下:
```
07:35:20
10:20:30
t1 is later than or equal to t2
```
希望我的回答能够帮助到您。
相关推荐
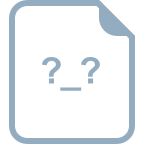
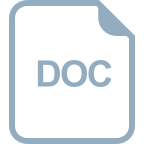














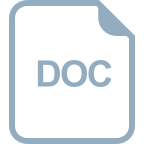