python+opencv锥桶识别框选代码
时间: 2023-10-02 08:05:19 浏览: 108
在锥桶识别的基础上,我们可以通过添加代码来实现框选锥桶的功能。以下是实现锥桶识别框选的代码:
```
import cv2
import numpy as np
img = cv2.imread('cone.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
blur = cv2.GaussianBlur(gray, (5, 5), 0)
edges = cv2.Canny(blur, 50, 150, apertureSize=3)
lines = cv2.HoughLines(edges, 1, np.pi/180, 100)
for line in lines:
rho, theta = line[0]
a = np.cos(theta)
b = np.sin(theta)
x0 = a * rho
y0 = b * rho
x1 = int(x0 + 1000 * (-b))
y1 = int(y0 + 1000 * (a))
x2 = int(x0 - 1000 * (-b))
y2 = int(y0 - 1000 * (a))
if abs(y2-y1) < 5:
cv2.line(img, (x1, y1), (x2, y2), (0, 0, 255), 2)
# 获取图像的宽和高
height, width = img.shape[:2]
# 创建一个空白图像,大小与原图像相同
mask = np.zeros((height, width), np.uint8)
# 多边形顶点坐标
pts = np.array([(0, height), (width*2/5, height*3/5), (width*3/5, height*3/5), (width, height)])
# 填充多边形
cv2.fillPoly(mask, [pts], (255, 255, 255))
# 将锥桶轮廓和多边形进行位运算
masked_img = cv2.bitwise_and(img, img, mask=mask)
# 将位运算结果转换为灰度图像
gray = cv2.cvtColor(masked_img, cv2.COLOR_BGR2GRAY)
# 二值化处理
ret, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY)
# 查找图像中的轮廓
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 遍历轮廓,框选锥桶
for cnt in contours:
x, y, w, h = cv2.boundingRect(cnt)
if w*h > 4000:
cv2.rectangle(img, (x, y), (x+w, y+h), (0, 255, 0), 2)
cv2.imshow('result', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
以上代码在锥桶识别的基础上添加了框选的部分。它首先创建一个与原图像大小相同的空白图像,然后在图像中绘制一个多边形,多边形的顶点坐标为锥桶的大致位置。接着,它将锥桶轮廓和多边形进行位运算,得到一个只包含锥桶的图像。然后,它将位运算结果转换为灰度图像,并进行二值化处理,得到锥桶的轮廓。最后,它遍历轮廓,框选锥桶,并在原图像中绘制矩形框。
相关推荐
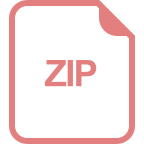
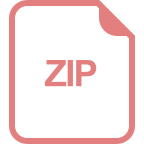
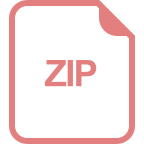














