jdbcTemplate.batchUpdate() 中public void add(User user) 的示例
时间: 2024-02-28 09:55:23 浏览: 23
Sure, here's an example of using `jdbcTemplate.batchUpdate()` with a `public void add(User user)` method:
```java
public void add(User user) {
String sql = "INSERT INTO users (id, name, age) VALUES (?, ?, ?)";
jdbcTemplate.update(sql, user.getId(), user.getName(), user.getAge());
}
public void batchInsert(List<User> users) {
String sql = "INSERT INTO users (id, name, age) VALUES (?, ?, ?)";
jdbcTemplate.batchUpdate(sql, new BatchPreparedStatementSetter() {
@Override
public void setValues(PreparedStatement ps, int i) throws SQLException {
User user = users.get(i);
ps.setInt(1, user.getId());
ps.setString(2, user.getName());
ps.setInt(3, user.getAge());
}
@Override
public int getBatchSize() {
return users.size();
}
});
}
```
In this example, we have two methods. The first method, `add(User user)`, inserts a single `User` object into the `users` table using the `jdbcTemplate.update()` method. The SQL query string is the same as in the previous example, and the parameter values are set using the `getId()`, `getName()`, and `getAge()` methods of the `User` object.
The second method, `batchInsert(List<User> users)`, inserts a batch of `User` objects into the `users` table using the `jdbcTemplate.batchUpdate()` method. This method is similar to the previous example, but instead of calling `jdbcTemplate.update()` for each `User` object, we're using a `BatchPreparedStatementSetter` object to set the parameter values for each row in the batch.
Both methods use the same SQL query string, so we don't need to repeat it. We can simply define it once at the beginning of the class.
相关推荐
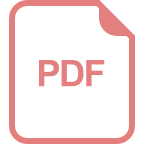














