把一个数组转换为链表,用Java实现
时间: 2024-02-28 16:52:54 浏览: 77
将一个数组转换为链表需要遍历整个数组,将数组元素的值存储到链表节点中。
以下是一个示例Java代码,假设链表节点的值类型为 int:
```java
class ListNode {
int val;
ListNode next;
ListNode(int val) {
this.val = val;
}
}
public class ArrayToLinkedList {
public static ListNode arrayToListNode(int[] arr) {
if (arr == null || arr.length == 0) {
return null;
}
ListNode head = new ListNode(arr[0]);
ListNode curr = head;
for (int i = 1; i < arr.length; i++) {
ListNode node = new ListNode(arr[i]);
curr.next = node;
curr = node;
}
return head;
}
public static void main(String[] args) {
int[] arr = {1, 2, 3, 4, 5};
ListNode head = arrayToListNode(arr);
// 遍历链表,输出节点值
ListNode curr = head;
while (curr != null) {
System.out.print(curr.val + " ");
curr = curr.next;
}
}
}
```
输出结果:
```
1 2 3 4 5
```
使用 `arrayToListNode` 方法可以将数组转换为链表。
阅读全文
相关推荐
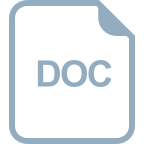
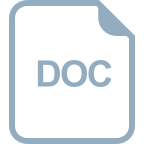
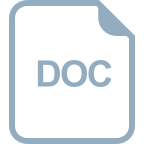
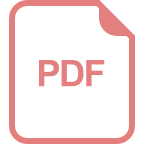
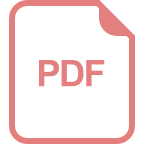
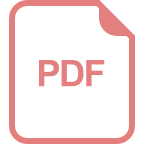

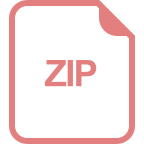
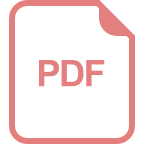
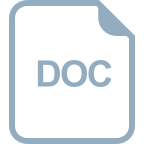
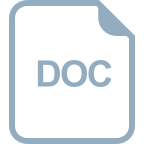
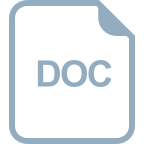
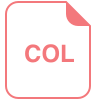
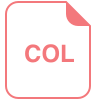
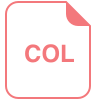
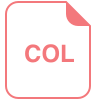
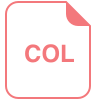
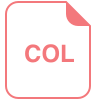
