给定前序和中序序列,设计算法,构建相应二叉树
时间: 2024-01-01 21:06:04 浏览: 61
给定二叉树的前序遍历和中序遍历,可以使用递归的方法构建出相应的二叉树。
具体的实现步骤如下:
1.从前序遍历中取出第一个节点,作为根节点。
2.在中序遍历中找到根节点的位置,将中序遍历分成左子树和右子树两部分。
3.根据左子树的长度,将前序遍历也分成左子树和右子树两部分。
4.递归构建左子树和右子树。
代码如下:
```python
class TreeNode:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
def buildTree(preorder, inorder):
if not preorder or not inorder:
return None
root_val = preorder.pop(0)
root_index = inorder.index(root_val)
root = TreeNode(root_val)
root.left = buildTree(preorder, inorder[:root_index])
root.right = buildTree(preorder, inorder[root_index+1:])
return root
```
其中,preorder和inorder分别代表前序遍历和中序遍历的列表。函数返回二叉树的根节点。
阅读全文
相关推荐
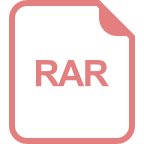
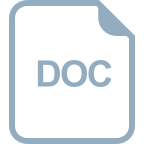
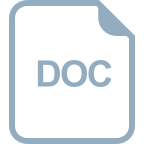
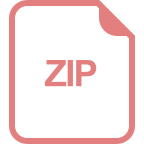
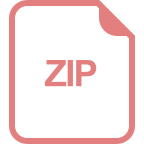
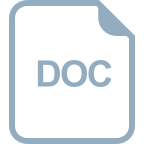
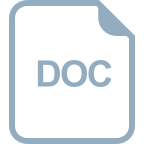
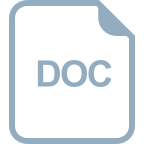
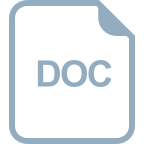
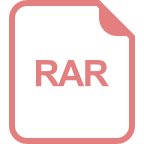
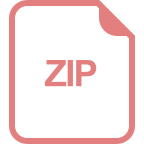
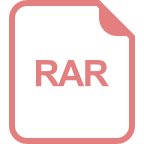
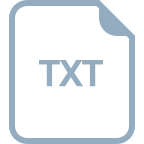
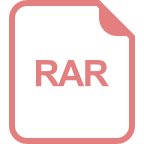
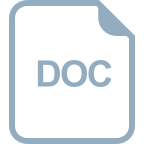
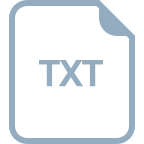