c语言建立链表尾插法
时间: 2023-12-21 12:31:20 浏览: 96
以下是使用C语言实现链表尾插法的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct Node {
int data;
struct Node* next;
};
// 尾插法建立链表
struct Node* createLinkedList() {
struct Node* head = NULL;
struct Node* tail = NULL;
int num;
printf("请输入链表元素(输入-1结束):\n");
while (1) {
scanf("%d", &num);
if (num == -1) {
break;
}
// 创建新节点
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = num;
newNode->next = NULL;
if (head == NULL) {
// 如果链表为空,将新节点设置为头节点和尾节点
head = newNode;
tail = newNode;
} else {
// 如果链表不为空,将新节点插入到尾节点后面,并更新尾节点
tail->next = newNode;
tail = newNode;
}
}
return head;
}
// 打印链表
void printLinkedList(struct Node* head) {
struct Node* current = head;
printf("链表元素为:");
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
int main() {
struct Node* head = createLinkedList();
printLinkedList(head);
return 0;
}
```
阅读全文
相关推荐
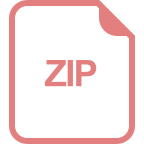





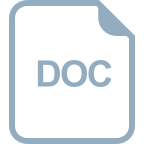








