结合IP获取的网卡信息,Java编程显示物理网卡的arp表
时间: 2024-10-15 18:19:19 浏览: 21
在Java中,获取物理网卡的ARP(地址解析协议)表通常涉及到操作系统级别的操作,因为ARP信息是在底层网络驱动和系统层面维护的。直接从Java程序访问ARP表通常是不可行的,因为它超出了Java应用的安全边界。不过,我们可以借助一些第三方库,如JNA (Java Native Access) 或 JNR (Java Native.Runtime),来间接地访问操作系统API。
以下是一个简单的示例,使用JNA尝试读取ARP表,但这并不是标准Java编程的一部分,而是一种高级技巧:
```java
import net.lingala.zip4j.core.IOUtils;
import com.sun.jna.Library;
import com.sun.jna.Native;
import com.sun.jna.platform.win32.Win32Library;
import com.sun.jna.ptr.IntByReference;
public class ARPTable {
private static final String ARP6432 = "iphlpapi.DLL";
private static final int G_ARPREQUESTINFO_SIZE = 80;
public static void main(String[] args) throws Exception {
Win32Library library = Native.loadLibrary(ARP6432, Library.class);
ArpFindEntry arpFindEntry = new ArpFindEntry();
ArpRequestInfo arpRequestInfo = new ArpRequestInfo();
// Initialize the ARP request info struct
arpRequestInfo.size = G_ARPREQUESTINFO_SIZE;
IntByReference resultLength = new IntByReference();
try {
library.ArpGetEntriesAtInterface(null, -1, null, 0, arpFindEntry, arpRequestInfo, resultLength);
} catch (Exception e) {
System.err.println("Error reading ARP table: " + e.getMessage());
return;
}
if (resultLength.getValue() > 0) {
byte[] buffer = new byte[resultLength.getValue()];
library.ArpGetEntriesAtInterface(null, -1, buffer, buffer.length, arpFindEntry, arpRequestInfo, resultLength);
// Process the ARP entries in the buffer here
// Note: This is a simplified example and does not include parsing or printing ARP entries
IOUtils.readFully(buffer, System.out);
} else {
System.out.println("No ARP entries found.");
}
}
// Define structures for API calls using JNA
public interface IPHLPAPI extends Win32Library {
// ... Declare ArpFindEntry and ArpRequestInfo structs here
}
@FunctionalInterface
interface ArpFindEntry extends Callback {
void callback(ArpRequestInfo arpRequestInfo);
}
@StructLayout(LayoutKind.Sequential)
public static class ArpRequestInfo extends Structure {
// ... Define fields like dwSize, pEntryBuffer, ulArpRequestCount, etc.
}
}
```
阅读全文
相关推荐
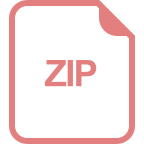
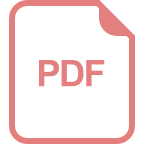
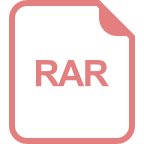

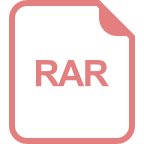
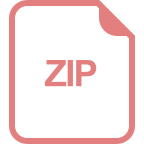
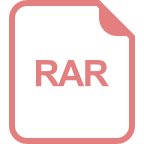
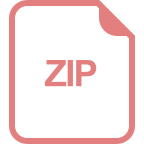
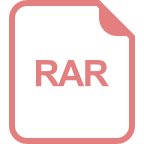
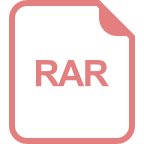
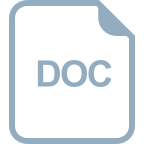
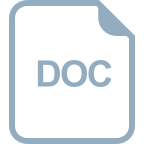
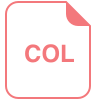
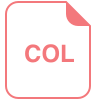
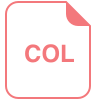
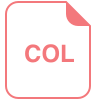
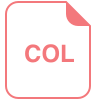
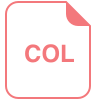
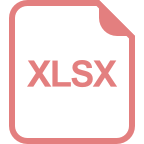
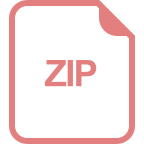