如何在C语言中实现一个基于有序数组的字典,并提供查找特定键值对的操作方法?
时间: 2024-11-04 15:22:46 浏览: 12
在C语言中实现一个基于有序数组的字典,需要考虑字典的插入、删除、查找等操作。在有序数组的上下文中,查找效率依赖于数组的有序性。以下是一个简化的实现方法,用于查找特定键值对:
参考资源链接:[数据结构:简单字典实现与应用](https://wenku.csdn.net/doc/34uxbmc81g?spm=1055.2569.3001.10343)
首先,定义一个键值对结构体以及字典结构体。键值对结构体用于存储键和值,而字典结构体则包含有序数组、键的比较函数指针以及记录数组中元素数量的变量。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义键值对结构体
typedef struct {
int key;
int value;
} KeyValuePair;
// 定义字典结构体
typedef struct {
KeyValuePair *arr;
int size;
int (*compare)(int a, int b);
} Dictionary;
// 键的比较函数,用于比较两个键
int compare_keys(int a, int b) {
return (a == b) ? 0 : (a > b) ? 1 : -1;
}
// 查找特定键的索引位置,使用二分查找法
int binary_search(Dictionary *dict, int key) {
int low = 0;
int high = dict->size - 1;
while (low <= high) {
int mid = low + (high - low) / 2;
int comp = dict->compare(dict->arr[mid].key, key);
if (comp == 0) {
return mid;
} else if (comp > 0) {
high = mid - 1;
} else {
low = mid + 1;
}
}
return -1; // 未找到
}
// 初始化字典
void init_dictionary(Dictionary *dict, int capacity) {
dict->arr = (KeyValuePair *)malloc(sizeof(KeyValuePair) * capacity);
dict->size = 0;
dict->compare = compare_keys;
}
// 插入键值对
void insert(Dictionary *dict, int key, int value) {
int index = binary_search(dict, key);
if (index >= 0) {
// 键已存在,更新值
dict->arr[index].value = value;
} else {
// 键不存在,插入新键值对
int i = -index - 1;
if (dict->size == capacity) {
// 扩容操作略
}
for (int j = dict->size; j > i; j--) {
dict->arr[j] = dict->arr[j - 1];
}
dict->arr[i].key = key;
dict->arr[i].value = value;
dict->size++;
}
}
// 查找特定键的值
int find(Dictionary *dict, int key) {
int index = binary_search(dict, key);
if (index >= 0) {
return dict->arr[index].value;
}
return -1; // 表示未找到
}
// 清理字典资源
void free_dictionary(Dictionary *dict) {
free(dict->arr);
dict->arr = NULL;
dict->size = 0;
}
int main() {
Dictionary dict;
init_dictionary(&dict, 10); // 假设初始容量为10
insert(&dict, 1, 100);
insert(&dict, 2, 200);
insert(&dict, 3, 300);
int value = find(&dict, 2);
if (value != -1) {
printf(
参考资源链接:[数据结构:简单字典实现与应用](https://wenku.csdn.net/doc/34uxbmc81g?spm=1055.2569.3001.10343)
阅读全文
相关推荐
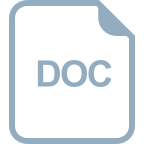
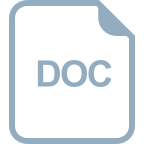
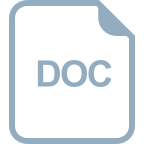
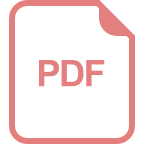
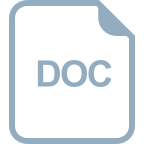
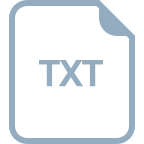
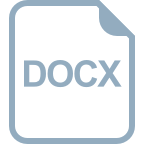
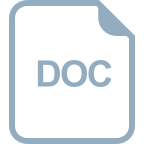
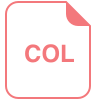
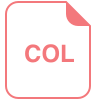
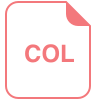
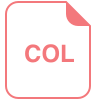
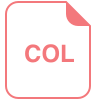
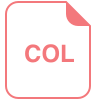
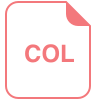
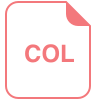
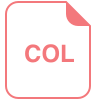
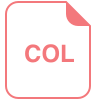
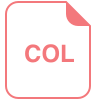