request.setAttribute("errorMsg", "用户名或密码错误"); request.getRequestDispatcher("login.jsp").forward(request, response);
时间: 2024-05-16 16:18:38 浏览: 13
这段代码主要涉及到 Java Web 开发中的 Servlet 技术。
`request.setAttribute("errorMsg", "用户名或密码错误");` 用于将错误信息存储在 request 对象中,其中 "errorMsg" 是属性名,"用户名或密码错误" 是属性值。
`request.getRequestDispatcher("login.jsp").forward(request, response);` 用于将请求转发到 login.jsp 页面,其中 request 和 response 分别代表请求和响应对象。这个方法会将请求和响应对象传递给 login.jsp 页面,让页面能够获取到传递的数据进行处理。
在 login.jsp 页面中,可以通过以下代码获取到存储在 request 对象中的错误信息并进行展示:
```
<%
String errorMsg = (String)request.getAttribute("errorMsg");
if (errorMsg != null) {
%>
<div class="error-msg"><%=errorMsg %></div>
<%
}
%>
```
上面的代码会首先获取存储在 request 对象中的 "errorMsg" 属性值,如果不为空则将其展示在页面上。
相关问题
利用EL和JSTL技术,改进用户登录页面(login.jsp),当用户信息验证成功则转到主页面(main,jsp)显示问候语,如“用户liming,你好!”,否则转回login.jsp,保留原来用户输入的用户名,并有提示信息:“用户名或密码错误,请重新输入”。
好的,针对您的问题,我可以提供以下解决方案:
1. 在login.jsp页面中添加表单,包括用户名和密码的输入框,以及“登录”按钮。
2. 在表单提交时,将输入的用户名和密码传递给后台进行验证。
3. 后台利用EL表达式和JSTL标签库获取表单中传递的用户名和密码,并与数据库中的数据进行比对。
4. 如果验证成功,则将用户名存入Session中,并跳转至main.jsp页面,利用EL表达式和JSTL标签库获取Session中的用户名,并在页面上显示问候语。
5. 如果验证失败,则返回login.jsp页面,并利用EL表达式和JSTL标签库在页面上显示错误提示信息和保留原来用户输入的用户名。
下面是示例代码(仅供参考,具体实现可能需要根据项目情况进行修改):
login.jsp页面代码:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>用户登录</title>
</head>
<body>
<h2>用户登录</h2>
<c:if test="${not empty errorMsg}">
<p style="color: red;">${errorMsg}</p>
</c:if>
<form method="post" action="login.do">
<p>
<label>用户名:</label><input type="text" name="username"
value="${not empty param.username ? param.username : ''}" />
</p>
<p>
<label>密码:</label><input type="password" name="password" />
</p>
<button type="submit">登录</button>
</form>
</body>
</html>
```
login.do后台代码:
```
// 获取用户输入的用户名和密码
String username = request.getParameter("username");
String password = request.getParameter("password");
// 进行验证
if ("admin".equals(username) && "123456".equals(password)) {
// 验证成功,将用户名存入Session中
HttpSession session = request.getSession();
session.setAttribute("username", username);
// 跳转至主页面
response.sendRedirect("main.jsp");
} else {
// 验证失败,返回登录页面,并显示错误提示信息和保留原来用户输入的用户名
request.setAttribute("errorMsg", "用户名或密码错误,请重新输入");
request.setAttribute("username", username);
request.getRequestDispatcher("login.jsp").forward(request, response);
}
```
main.jsp页面代码:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>主页面</title>
</head>
<body>
<h2>主页面</h2>
<p>用户<c:out value="${sessionScope.username}" />,你好!</p>
</body>
</html>
```
使用servlet实现用户登陆---用户名密码错误--带着错误信息提示跳转回登陆页面给用户一个提示(转发到登陆页面)
好的,我来为您详细解答一下。
首先,在用户登陆时,需要在后台对输入的用户名和密码进行验证。如果验证不通过,就需要返回一个错误信息并将用户重定向回登陆页面。
以下是一个使用Servlet实现用户登陆的示例代码:
```java
@WebServlet("/login")
public class LoginServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String username = request.getParameter("username");
String password = request.getParameter("password");
if (username.equals("admin") && password.equals("123456")) {
// 登陆成功
response.sendRedirect("welcome.jsp");
} else {
// 登陆失败,返回错误信息并重定向回登陆页面
request.setAttribute("errorMsg", "用户名或密码错误!");
RequestDispatcher dispatcher = request.getRequestDispatcher("login.jsp");
dispatcher.forward(request, response);
}
}
}
```
在上述代码中,我们首先获取用户输入的用户名和密码。如果用户名和密码都是正确的,我们就使用`response.sendRedirect()`方法将用户重定向到欢迎页面。
如果用户名或密码不正确,我们就可以使用`request.setAttribute()`方法将错误信息设置到请求的属性中。然后,使用`RequestDispatcher`的`forward()`方法将请求转发回登陆页面,并在登陆页面中显示错误信息。
在登陆页面中,我们可以使用EL表达式`${errorMsg}`来获取错误信息并显示给用户。
下面是一个简单的登陆页面示例代码:
```html
<html>
<head>
<title>Login</title>
</head>
<body>
<h1>Login</h1>
<form action="login" method="post">
<div>
<label for="username">Username:</label>
<input type="text" id="username" name="username"/>
</div>
<div>
<label for="password">Password:</label>
<input type="password" id="password" name="password"/>
</div>
<div>
<input type="submit" value="Login"/>
</div>
<div style="color:red">${errorMsg}</div>
</form>
</body>
</html>
```
在上述代码中,我们使用`<form>`元素将用户输入的用户名和密码提交到`LoginServlet`中进行验证。如果验证失败,错误信息将显示在`<div>`元素中。
希望这个示例代码可以帮助到您,如果您还有其他问题,请随时提出,我会尽力回答。
相关推荐
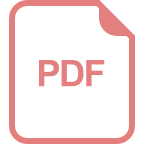
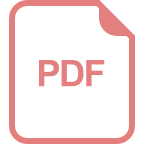
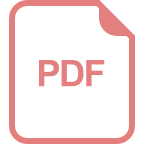










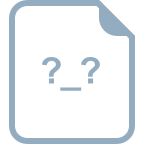
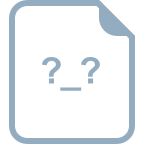
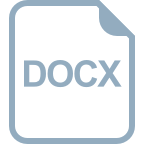