#include <stdio.h> #include <stdlib.h> struct student { long num; struct student *next; }; struct student *createlink(int); struct student *createnode(); void del(struct student *,struct student *); void output(struct student *); main() { int an=5,bn=3; struct student *ahead,*bhead; ahead=(struct student *)malloc (sizeof(struct student)); bhead=(struct student *)malloc (sizeof(struct student)); ahead->next=createlink(an); bhead->next=createlink(bn); del(ahead,bhead); output(ahead); } void del(struct student *ahead,struct student *bhead) { } void output(struct student *head) { struct student *p=head->next; while(p!=NULL) { printf("%ld\n",p->num); p=p->next; } } struct student *createlink(int n) { int i; struct student *head,*p1,*p2; for(i=0;i<n;i++) { if(i==0) head=p1=createnode(); else { p2=createnode(); p1->next=p2; p1=p2; } } return(head); } struct student *createnode() { struct student *p; p=(struct student *)malloc (sizeof(struct student)); printf("Please:"); scanf("%ld",&p->num); p->next=NULL; return(p); }
时间: 2024-03-18 20:43:42 浏览: 71
这段代码定义了一个`student`结构体,包含学号和指向下一个结构体的指针。然后通过`createlink`函数创建链表,该函数返回一个指向头结点的指针。`createlink`函数接受一个参数n,表示创建链表的长度。内部使用`createnode`函数创建每个结点,并将它们连接起来。`createnode`函数接受用户输入的学号并返回一个指向新结点的指针。
主函数中创建了两个链表,分别表示A和B两个集合。然后调用`del`函数删除A和B中重复的元素。最后调用`output`函数输出结果。
需要注意的是,`del`函数的内容被省略了,因此这段代码并不能正常运行。
相关问题
#include <stdio.h> #include <windows.h> #include <stdlib.h> #define LEN sizeof(struct Student)
这段代码包含了三个常用的C语言头文件:`stdio.h`用于输入输出操作,`windows.h`通常与Windows API交互相关,而`stdlib.h`提供了标准库函数,如内存管理。
`#define LEN sizeof(struct Student)` 是一个预处理器宏定义,它将计算`struct Student`结构体的大小,并将其赋值给名为LEN的常量。这样,在程序中可以直接使用`LEN`代替`sizeof(struct Student)`来避免在每个使用该结构体大小的地方重复计算。
结构体(struct)`Student`没有在这里直接定义,所以我们不清楚它的具体内容。如果`struct Student`中有变量或成员函数,它的大小会包括这些元素的总和。
#include<stdio.h>#include<string.h>#include<stdlib.h>struct+stu+{ char++data[12]; struct+stu++*next;
这段代码定义了一个结构体`stu`,其中包含一个名为`data`的字符数组和一个指向下一个`stu`结构体的指针`next`。这个结构体可以用来创建一个链表,每个节点都是一个`stu`结构体,`data`数组可以存储一些数据,`next`指针指向下一个节点。这个链表可以用来实现一些数据结构,例如队列、栈等。
阅读全文
相关推荐
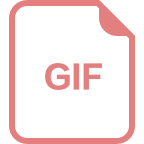













