写一个java的快速排序的代码
时间: 2023-08-31 16:43:29 浏览: 110
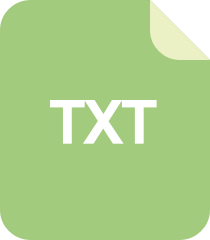
快速排序 java代码
### 回答1:
public class QuickSort {
public static void sort(int[] arr, int left, int right) {
if (left < right) {
int pivot = partition(arr, left, right);
sort(arr, left, pivot - 1);
sort(arr, pivot + 1, right);
}
}
public static int partition(int[] arr, int left, int right) {
int pivot = arr[right];
int i = left - 1;
for (int j = left; j < right; j++) {
if (arr[j] <= pivot) {
i++;
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
int temp = arr[i + 1];
arr[i + 1] = arr[right];
arr[right] = temp;
return (i + 1);
}
}
### 回答2:
快速排序是一种常用的排序算法,它基于分治法的思想。该算法采用了递归的方式,将待排序的数组分成两个子数组,然后对这两个子数组分别进行排序,最后将两个有序的子数组合并成一个有序的数组。
下面是一个实现快速排序的Java代码示例:
```java
public class QuickSort {
public static void main(String[] args) {
int[] arr = {9, 5, 1, 3, 7, 6, 2, 8, 4};
quickSort(arr, 0, arr.length - 1);
for (int num : arr) {
System.out.print(num + " ");
}
}
public static void quickSort(int[] arr, int low, int high) {
if (low < high) {
int pivotIndex = partition(arr, low, high);
quickSort(arr, low, pivotIndex - 1);
quickSort(arr, pivotIndex + 1, high);
}
}
public static int partition(int[] arr, int low, int high) {
int pivot = arr[low];
while (low < high) {
while (low < high && arr[high] >= pivot) {
high--;
}
arr[low] = arr[high];
while (low < high && arr[low] <= pivot) {
low++;
}
arr[high] = arr[low];
}
arr[low] = pivot;
return low;
}
}
```
以上代码中,`quickSort`方法是快速排序的入口,它将待排序数组的首尾索引传入。在`quickSort`方法中,使用`partition`方法将数组分割成两部分,左边部分的元素都小于等于基准值,右边部分的元素都大于等于基准值。然后对两部分分别递归调用`quickSort`方法,直到只剩下一个元素。
`partition`方法中,首先选择一个基准值,然后使用两个指针从数组的首尾开始向中间遍历。指针向中间移动时,如果找到了比基准值小的元素或比基准值大的元素,就将它们交换位置。当两个指针相遇时,将基准值交换到相遇点的位置,并返回相遇点的索引作为下一次划分的依据。
以上代码实现了快速排序算法,可以对任意长度的整数数组进行排序。输出结果为排序后的数组:1 2 3 4 5 6 7 8 9。
### 回答3:
快速排序(Quick Sort)是一种常用的排序算法,基于分治思想。它的基本思想是选择一个基准元素,将数组分成两个子数组,分别是小于基准元素的部分和大于基准元素的部分,然后递归地对这两个子数组进行排序。
以下是用Java实现的快速排序代码:
```java
public class QuickSort {
public static void main(String[] args) {
int[] array = {6, 2, 3, 9, 5, 1, 8, 7, 4};
quickSort(array, 0, array.length - 1);
System.out.println("排序结果:");
for (int num : array) {
System.out.print(num + " ");
}
}
public static void quickSort(int[] array, int left, int right) {
if (left < right) {
int partitionIndex = partition(array, left, right);
// 对分区后的子数组进行递归排序
quickSort(array, left, partitionIndex - 1);
quickSort(array, partitionIndex + 1, right);
}
}
public static int partition(int[] array, int left, int right) {
int pivot = array[right]; // 选择最右边的元素作为基准
int i = left - 1;
for (int j = left; j < right; j++) {
if (array[j] < pivot) {
i++;
swap(array, i, j);
}
}
swap(array, i + 1, right);
return i + 1;
}
public static void swap(int[] array, int i, int j) {
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}
```
该代码先定义了一个QuickSort类,然后在main方法中定义了一个待排序的数组。接着调用quickSort方法对数组进行快速排序,并输出排序结果。quickSort方法负责递归地排序子数组,而partition方法则负责对当前子数组进行划分操作,将小于基准的元素移动到基准的左边,大于基准的元素移动到基准的右边。swap方法用于交换数组中的两个元素。
快速排序是一种高效的排序算法,平均时间复杂度为O(nlogn),最坏情况下的时间复杂度为O(n^2),空间复杂度为O(logn)。
阅读全文
相关推荐
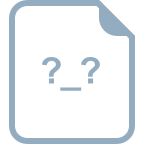
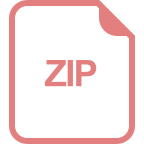
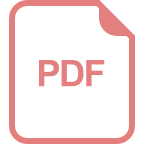
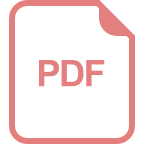
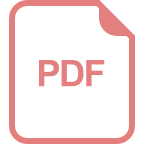
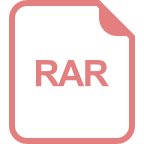
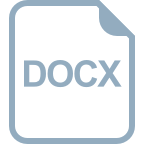
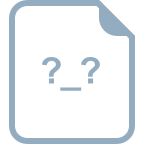
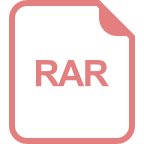
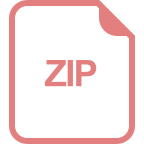
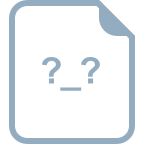