c++中一个完整的团购订单信息管理系统。该系现团购订单信息的添加、查找、修改、删除、浏览等功能。要求输入登录口令,口令正确可以继续操作。用结构体数组实现。
时间: 2023-08-11 18:05:43 浏览: 77
好的,下面是一个简单的实现:
```c++
#include <iostream>
#include <string>
using namespace std;
// 定义订单结构体
struct Order {
string orderID; // 订单编号
string customerName; // 客户名称
string goodsName; // 商品名称
double price; // 商品单价
int quantity; // 商品数量
};
// 定义订单管理类
class OrderManager {
private:
Order orders[100]; // 存储订单的结构体数组
int count; // 当前订单数量
string password; // 登录口令
public:
// 构造函数,初始化订单数量为0,并设置登录口令为123456
OrderManager() {
count = 0;
password = "123456";
}
// 添加订单
void addOrder(string id, string name, string goods, double price, int quantity) {
orders[count].orderID = id;
orders[count].customerName = name;
orders[count].goodsName = goods;
orders[count].price = price;
orders[count].quantity = quantity;
count++;
cout << "订单添加成功!" << endl;
}
// 查找订单
void findOrder(string id) {
for (int i = 0; i < count; i++) {
if (orders[i].orderID == id) {
cout << "订单编号:" << orders[i].orderID << endl;
cout << "客户名称:" << orders[i].customerName << endl;
cout << "商品名称:" << orders[i].goodsName << endl;
cout << "商品单价:" << orders[i].price << endl;
cout << "商品数量:" << orders[i].quantity << endl;
return;
}
}
cout << "未找到订单!" << endl;
}
// 修改订单
void modifyOrder(string id, string name, string goods, double price, int quantity) {
for (int i = 0; i < count; i++) {
if (orders[i].orderID == id) {
orders[i].customerName = name;
orders[i].goodsName = goods;
orders[i].price = price;
orders[i].quantity = quantity;
cout << "订单修改成功!" << endl;
return;
}
}
cout << "未找到订单!" << endl;
}
// 删除订单
void deleteOrder(string id) {
for (int i = 0; i < count; i++) {
if (orders[i].orderID == id) {
for (int j = i; j < count - 1; j++) {
orders[j] = orders[j + 1];
}
count--;
cout << "订单删除成功!" << endl;
return;
}
}
cout << "未找到订单!" << endl;
}
// 浏览所有订单
void viewAllOrders() {
for (int i = 0; i < count; i++) {
cout << "订单编号:" << orders[i].orderID << endl;
cout << "客户名称:" << orders[i].customerName << endl;
cout << "商品名称:" << orders[i].goodsName << endl;
cout << "商品单价:" << orders[i].price << endl;
cout << "商品数量:" << orders[i].quantity << endl;
cout << endl;
}
}
// 登录
bool login(string pwd) {
if (pwd == password) {
return true;
}
else {
return false;
}
}
};
int main() {
OrderManager manager; // 创建订单管理对象
string password; // 用户输入的口令
string id, name, goods; // 订单编号、客户名称、商品名称
double price; // 商品单价
int quantity; // 商品数量
int option; // 用户选择的功能
cout << "请输入登录口令:" << endl;
cin >> password;
if (!manager.login(password)) {
cout << "登录失败!" << endl;
return 0;
}
while (true) {
cout << "请选择功能:" << endl;
cout << "1. 添加订单" << endl;
cout << "2. 查找订单" << endl;
cout << "3. 修改订单" << endl;
cout << "4. 删除订单" << endl;
cout << "5. 浏览所有订单" << endl;
cout << "6. 退出系统" << endl;
cin >> option;
switch (option) {
case 1:
cout << "请输入订单编号、客户名称、商品名称、商品单价、商品数量:" << endl;
cin >> id >> name >> goods >> price >> quantity;
manager.addOrder(id, name, goods, price, quantity);
break;
case 2:
cout << "请输入要查找的订单编号:" << endl;
cin >> id;
manager.findOrder(id);
break;
case 3:
cout << "请输入要修改的订单编号、客户名称、商品名称、商品单价、商品数量:" << endl;
cin >> id >> name >> goods >> price >> quantity;
manager.modifyOrder(id, name, goods, price, quantity);
break;
case 4:
cout << "请输入要删除的订单编号:" << endl;
cin >> id;
manager.deleteOrder(id);
break;
case 5:
manager.viewAllOrders();
break;
case 6:
return 0;
default:
cout << "无效的选项!" << endl;
break;
}
}
return 0;
}
```
这个程序中,我们使用了结构体数组来存储订单信息,并用一个计数器变量来记录当前订单数量。订单管理类中定义了添加、查找、修改、删除、浏览等功能,其中查找、修改、删除都需要遍历结构体数组来查找对应的订单。登录功能使用了一个简单的口令验证机制。在主函数中,我们使用一个循环来让用户选择不同的功能,直到用户选择退出系统为止。
相关推荐
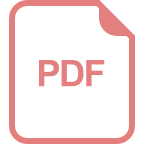
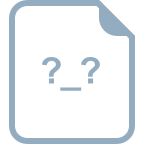














