如何使用epoll机制实现ubus下的notify发送消息和register接受消息 写出代码
时间: 2024-03-10 21:46:18 浏览: 139
下面是一个使用 epoll 机制实现 ubus 下的 notify 发送消息和 register 接收消息的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/epoll.h>
#include <libubox/blobmsg_json.h>
#include <libubus.h>
#define MAX_EVENTS 10
int main(int argc, char *argv[]) {
int epoll_fd, ubus_fd, n;
struct epoll_event event, events[MAX_EVENTS];
struct ubus_context *ctx;
struct ubus_request req;
// 初始化 ubus 对象
ctx = ubus_connect(NULL);
if (!ctx) {
fprintf(stderr, "Failed to connect to ubus\n");
return -1;
}
// 创建 epoll 对象
epoll_fd = epoll_create1(0);
if (epoll_fd == -1) {
fprintf(stderr, "Failed to create epoll object\n");
ubus_free(ctx);
return -1;
}
// 注册消息接收回调函数
ubus_fd = ubus_get_fd(ctx);
event.events = EPOLLIN;
event.data.fd = ubus_fd;
if (epoll_ctl(epoll_fd, EPOLL_CTL_ADD, ubus_fd, &event) == -1) {
fprintf(stderr, "Failed to register ubus_fd to epoll\n");
close(epoll_fd);
ubus_free(ctx);
return -1;
}
// 发送消息
memset(&req, 0, sizeof(req));
req.path = "/ubus/path";
req.method = "method_name";
req.args = blobmsg_new_array();
blobmsg_add_string(req.args, NULL, "arg1");
blobmsg_add_string(req.args, NULL, "arg2");
ubus_invoke_async(ctx, &req, &req.req_id);
event.events = EPOLLIN;
event.data.fd = req.req_id;
if (epoll_ctl(epoll_fd, EPOLL_CTL_ADD, req.req_id, &event) == -1) {
fprintf(stderr, "Failed to register request event to epoll\n");
close(epoll_fd);
ubus_free(ctx);
return -1;
}
// 等待事件发生并处理事件
while (1) {
n = epoll_wait(epoll_fd, events, MAX_EVENTS, -1);
if (n == -1) {
fprintf(stderr, "Failed to wait for events\n");
close(epoll_fd);
ubus_free(ctx);
return -1;
}
for (int i = 0; i < n; i++) {
if (events[i].data.fd == ubus_fd) {
ubus_handle_event(ctx);
} else {
ubus_complete_request(ctx, events[i].data.fd);
}
}
}
// 释放资源
blobmsg_free(req.args);
close(epoll_fd);
ubus_free(ctx);
return 0;
}
```
以上代码仅供参考,具体实现方式需要根据具体需求进行调整。
阅读全文
相关推荐
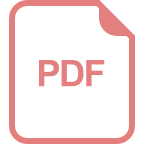
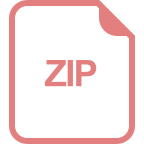
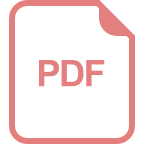


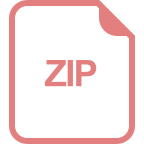
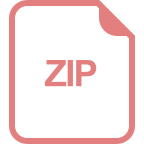
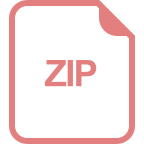
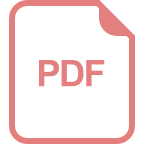
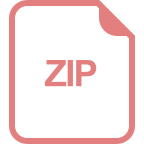
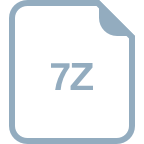
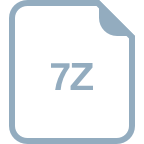
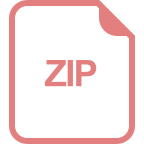
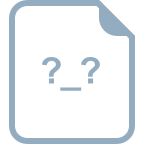