解释下列代码import java.io.BufferedReader; import java.io.File; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.IOException; import java.io.InputStreamReader; import java.io.PrintStream;
时间: 2023-10-10 14:13:14 浏览: 241
这段代码是 Java 语言的导入语句,它们将 Java 标准库中的 IO(Input/Output)包中的一些类和接口导入到当前代码文件中,以便在代码中使用这些类和接口。
具体来说,代码中使用了以下类和接口:
- BufferedReader:这个类用于从字符输入流中读取文本,它提供了一些方法来读取字符、行、文本等。
- File:这个类用于表示文件或目录的路径名,它可以用于创建、删除、重命名、判断文件或目录是否存在等操作。
- FileNotFoundException:这个异常表示找不到文件或目录的异常,当尝试打开一个不存在的文件或目录时,会抛出这个异常。
- FileReader:这个类用于从文件中读取字符流,它是 BufferedReader 类的子类。
- IOException:这个异常表示 IO 操作可能会引发的异常,如读/写文件、网络连接等操作。
- InputStreamReader:这个类用于将字节输入流转换为字符输入流,它提供了一些方法来读取字符。
- PrintStream:这个类用于将数据格式化为文本输出到输出流中,它提供了一些方法来打印输出。
在这段代码中,可能会在后续的代码中使用这些类和接口来读取文件或从标准输入读取数据,并将数据输出到控制台或文件中。
相关问题
根据以下要求:Instead of using a text file to save all the point coordinates one by one, change the savaData method and the constructor of the Model class to use object serialization and a binary file called "points.bin" to write / read the whole arraylist of points to / from the file in one operation.修改下述代码:import java.awt.Point; import java.io.BufferedReader; import java.io.BufferedWriter; import java.io.File; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.FileWriter; import java.io.IOException; import java.io.PrintWriter; import java.util.ArrayList; import java.util.Scanner; public class Model { private ArrayList<Point> points; private ArrayList<ModelListener> listeners; public Model() { points = new ArrayList<Point>(); listeners = new ArrayList<ModelListener>(); // Read points from file if it exists File file = new File("points.txt"); if (file.exists()) { try { Scanner scanner = new Scanner(file); while (scanner.hasNextLine()) { String[] coordinates = scanner.nextLine().split(" "); int x = (int) Double.parseDouble(coordinates[0]); int y = (int) Double.parseDouble(coordinates[1]); points.add(new Point(x, y)); } scanner.close(); } catch (FileNotFoundException e) { e.printStackTrace(); } } } public void addListener(ModelListener l) { listeners.add(l); } public ArrayList<Point> getPoints() { return points; } public void addPoint(Point p) { points.add(p); notifyListeners(); // points changed so notify the listeners. saveData(); // save point to file } public void clearAllPoints() { points.clear(); notifyListeners(); // points changed so notify the listeners. saveData(); // save empty list to file } public void deleteLastPoint() { if (points.size() > 0) { points.remove(points.size() - 1); notifyListeners(); // points changed so notify the listeners. saveData(); // save updated list to file } } private void notifyListeners() { for (ModelListener l : listeners) { l.update(); // Tell the listener that something changed. } } public int numberOfPoints() { return points.size(); } public void saveData() { try { FileWriter writer = new FileWriter("points.txt"); for (Point p : points) { writer.write(p.x + " " + p.y + "\n"); } writer.close(); } catch (IOException e) { e.printStackTrace(); } }
以下是修改后的代码:
import java.awt.Point;
import java.io.*;
public class Model implements Serializable {
private ArrayList<Point> points;
private ArrayList<ModelListener> listeners;
private static final String FILE_NAME = "points.bin";
public Model() {
points = new ArrayList<Point>();
listeners = new ArrayList<ModelListener>();
// Read points from file if it exists
File file = new File(FILE_NAME);
if (file.exists()) {
try {
ObjectInputStream in = new ObjectInputStream(new FileInputStream(file));
points = (ArrayList<Point>) in.readObject();
in.close();
} catch (IOException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
public void addListener(ModelListener l) {
listeners.add(l);
}
public ArrayList<Point> getPoints() {
return points;
}
public void addPoint(Point p) {
points.add(p);
notifyListeners(); // points changed so notify the listeners.
saveData(); // save point to file
}
public void clearAllPoints() {
points.clear();
notifyListeners(); // points changed so notify the listeners.
saveData(); // save empty list to file
}
public void deleteLastPoint() {
if (points.size() > 0) {
points.remove(points.size() - 1);
notifyListeners(); // points changed so notify the listeners.
saveData(); // save updated list to file
}
}
private void notifyListeners() {
for (ModelListener l : listeners) {
l.update(); // Tell the listener that something changed.
}
}
public int numberOfPoints() {
return points.size();
}
public void saveData() {
try {
ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream(FILE_NAME));
out.writeObject(points);
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
这里我们使用了Java的序列化机制来保存整个ArrayList对象,同时将数据保存到了一个二进制文件中(名为"points.bin")。在构造函数中,我们首先检查是否存在该文件,如果存在,则通过ObjectInputStream读取该文件并将ArrayList对象恢复到程序中。在saveData()方法中,我们使用ObjectOutputStream将整个ArrayList对象写入文件。注意:我们还必须将Model类实现Serializable接口来支持序列化。
通过带缓冲区的 java.io.FileReader 类实现从已有的文本文件“d:\exam.txt”中读出内容, 并打印在控制台。
可以使用以下代码实现:
```java
import java.io.BufferedReader;
import java.io.FileReader;
public class FileReadingExample {
public static void main(String[] args) {
try {
BufferedReader reader = new BufferedReader(new FileReader("d:\\exam.txt"));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
reader.close();
} catch (Exception e) {
System.out.println("Error occurred while reading the file.");
e.printStackTrace();
}
}
}
```
解析:
- 首先,我们创建了一个 `BufferedReader` 类的对象,该对象使用 `FileReader` 类的对象来读取文件。`FileReader` 类是一个用于读取字符文件的便捷类。
- 我们使用 `while` 循环逐行读取文件内容,直到读取完所有行。`readLine()` 方法返回一个包含当前行内容的字符串。
- 最后,我们关闭了 `BufferedReader` 对象,以释放资源并避免内存泄漏。
注意:在使用 FileReader 时可能会抛出 FileNotFoundException 异常,因此需要在 try-catch 块中处理它。
阅读全文
相关推荐
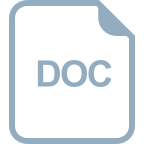
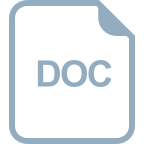
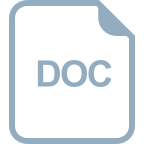
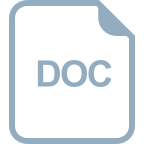
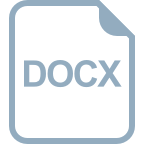
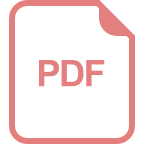
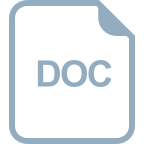
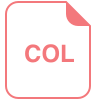
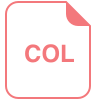
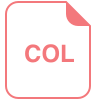
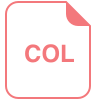
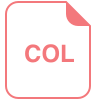
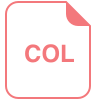
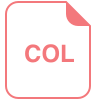
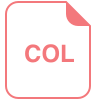


