react 将 普通的 loading 组件封装成 hook 调用
时间: 2023-07-16 21:17:05 浏览: 85
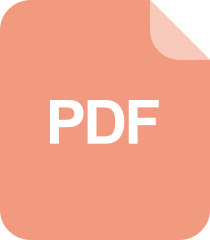
react-hooks如何使用?
好的,以下是一个简单的示例:
```javascript
import { useState, useEffect } from "react";
const useLoading = (loadingComponent) => {
const [isLoading, setIsLoading] = useState(false);
useEffect(() => {
return () => {
setIsLoading(false);
};
}, []);
const showLoading = () => {
setIsLoading(true);
};
const hideLoading = () => {
setIsLoading(false);
};
const LoadingIndicator = () => {
return isLoading ? loadingComponent : null;
};
return [LoadingIndicator, showLoading, hideLoading];
};
export default useLoading;
```
这个 hook 接受一个 loading 组件作为参数,返回一个数组,包含了一个 LoadingIndicator 组件和两个函数 showLoading 和 hideLoading。
使用示例:
```javascript
import React from "react";
import useLoading from "./useLoading";
import Loading from "./Loading";
const MyComponent = () => {
const [LoadingIndicator, showLoading, hideLoading] = useLoading(
<Loading />
);
const handleClick = () => {
showLoading();
// 模拟一个异步操作
setTimeout(() => {
hideLoading();
}, 2000);
};
return (
<div>
<button onClick={handleClick}>点击加载</button>
<LoadingIndicator />
</div>
);
};
export default MyComponent;
```
在上面的示例中,我们使用 useLoading hook 将一个普通的 Loading 组件封装成了一个可以在 MyComponent 组件中调用的 LoadingIndicator 和 showLoading/hideLoading 函数。在 handleClick 函数中,我们调用 showLoading 函数显示 LoadingIndicator,然后模拟一个异步操作,2s 后调用 hideLoading 函数隐藏 LoadingIndicator。
阅读全文
相关推荐
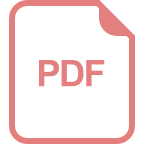
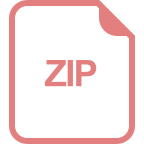
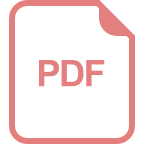
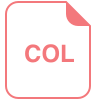
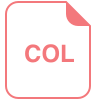
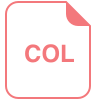
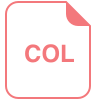
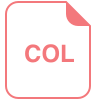
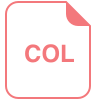
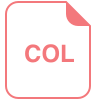
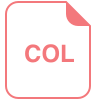
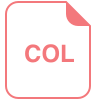
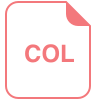
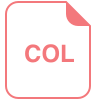
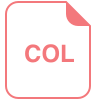
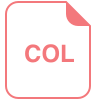
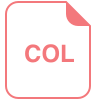