qt5多线程下,主线程同一时刻调用所有子线程的函数
时间: 2023-08-04 20:13:36 浏览: 59
在 Qt5 中,可以使用信号和槽机制实现主线程调用所有子线程的函数。
首先,你需要在主线程中创建所有子线程的实例,并为每个子线程定义一个信号和一个槽。
```cpp
class MyThread : public QThread {
Q_OBJECT
signals:
void mySignal();
public slots:
void mySlot();
};
```
然后,在主线程中连接每个子线程的信号到主线程的槽。
```cpp
MyThread thread1, thread2, thread3;
connect(&thread1, SIGNAL(mySignal()), this, SLOT(mySlot()));
connect(&thread2, SIGNAL(mySignal()), this, SLOT(mySlot()));
connect(&thread3, SIGNAL(mySignal()), this, SLOT(mySlot()));
```
接下来,在每个子线程的执行函数中,发射信号。
```cpp
void MyThread::run() {
// do some work
emit mySignal();
}
```
最后,在主线程中启动每个子线程,并等待它们完成。
```cpp
thread1.start();
thread2.start();
thread3.start();
thread1.wait();
thread2.wait();
thread3.wait();
```
当子线程执行完成并发射信号时,主线程的槽函数会被调用。在槽函数中,你可以执行所有子线程共享的代码。
相关推荐
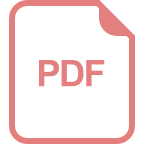
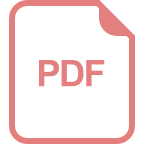














