qt在多线程中如何主线程如何向子线程传参
时间: 2023-11-16 19:32:47 浏览: 823
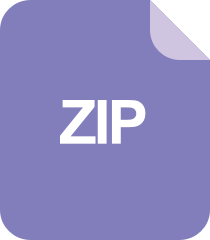
Qt线程和主线程传递数据
在Qt中,主线程可以通过以下方式向子线程传递参数:
1. 使用信号与槽机制:在主线程中定义一个信号,子线程中定义一个槽函数,主线程通过emit发送信号并传递参数。
2. 使用QThread的构造函数:在创建子线程的时候,可以在构造函数中传递参数。
3. 使用QRunnable:将需要传递的参数打包成一个类,继承自QRunnable,然后将其传递给QThreadPool来执行。
下面是使用信号与槽机制的示例代码:
```cpp
// MyThread.h
#include <QThread>
class MyThread : public QThread
{
Q_OBJECT
public:
explicit MyThread(QObject *parent = nullptr);
signals:
void sendData(QString data);
protected:
void run() override;
};
// MyThread.cpp
#include "MyThread.h"
#include <QDebug>
MyThread::MyThread(QObject *parent) : QThread(parent)
{
}
void MyThread::run()
{
// 执行子线程任务
QString data = "Hello, World!"; // 子线程要传递的数据
emit sendData(data); // 发送信号并传递数据
}
// MainWindow.h
#include <QMainWindow>
#include "MyThread.h"
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private slots:
void on_recvData(QString data);
private:
Ui::MainWindow *ui;
MyThread *m_thread;
};
// MainWindow.cpp
#include "MainWindow.h"
#include "ui_MainWindow.h"
#include <QDebug>
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
{
ui->setupUi(this);
m_thread = new MyThread(this);
connect(m_thread, &MyThread::sendData, this, &MainWindow::on_recvData); // 连接信号与槽函数
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::on_recvData(QString data)
{
qDebug() << "Received data from sub-thread: " << data;
}
void MainWindow::on_pushButton_clicked()
{
m_thread->start(); // 启动子线程
}
```
在上面的代码中,主线程通过connect连接了MyThread的sendData信号和MainWindow的on_recvData槽函数,当MyThread发送sendData信号时,主线程会自动调用on_recvData槽函数,并将数据作为参数传递进来。
阅读全文
相关推荐
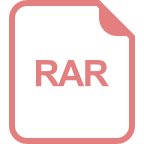
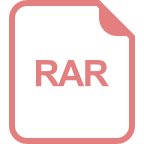
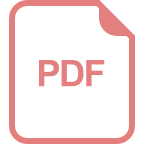
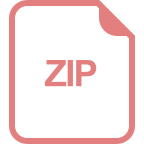
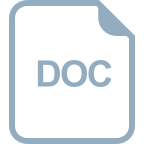
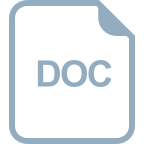









