linux i2c读写eeprom
时间: 2023-04-24 13:00:03 浏览: 367
要在Linux中读写I2C EEPROM,需要使用i2c-tools软件包中的工具。首先,需要确定EEPROM的I2C地址和总线号。可以使用命令“i2cdetect -l”列出所有可用的I2C总线,然后使用命令“i2cdetect -y <bus number>”扫描指定的总线,以查找连接的设备及其地址。
一旦确定了EEPROM的地址和总线号,就可以使用命令“i2cget”读取EEPROM中的数据,或使用命令“i2cset”写入数据。例如,要读取EEPROM中地址为x10的字节,可以使用命令“i2cget -y <bus number> <eeprom address> x10”,其中“<bus number>”是总线号,“<eeprom address>”是EEPROM的I2C地址。
要写入数据到EEPROM中,可以使用命令“i2cset -y <bus number> <eeprom address> <offset> <value>”,其中“<offset>”是要写入的EEPROM地址,而“<value>”是要写入的数据。请注意,EEPROM通常有一个写保护引脚,需要在写入数据之前将其禁用。
相关问题
linux i2c读写eeprom代码
以下是一个简单的 Linux C 代码示例,用于通过 I2C 接口读写 EEPROM:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/ioctl.h>
#include <linux/i2c-dev.h>
#define EEPROM_ADDR 0x50
#define EEPROM_SIZE 256
int main()
{
int file;
char filename[20];
char data[EEPROM_SIZE];
int i;
sprintf(filename, "/dev/i2c-1");
if ((file = open(filename, O_RDWR)) < 0) {
perror("Failed to open the i2c bus");
exit(1);
}
if (ioctl(file, I2C_SLAVE, EEPROM_ADDR) < 0) {
perror("Failed to acquire bus access and/or talk to slave");
exit(1);
}
// 读取 EEPROM 数据
if (read(file, data, EEPROM_SIZE) != EEPROM_SIZE) {
perror("Failed to read from the i2c bus");
} else {
printf("Read data from EEPROM:\n");
for (i = 0; i < EEPROM_SIZE; i++) {
printf("%02x ", data[i]);
if ((i + 1) % 16 == 0) printf("\n");
}
}
// 写入 EEPROM 数据
for (i = 0; i < EEPROM_SIZE; i++) {
data[i] = i;
}
if (write(file, data, EEPROM_SIZE) != EEPROM_SIZE) {
perror("Failed to write to the i2c bus");
} else {
printf("Wrote data to EEPROM\n");
}
close(file);
return 0;
}
```
在上面的代码中,我们首先打开 /dev/i2c-1 设备节点,这是 I2C 接口的默认节点。然后我们使用 ioctl 函数设置从设备地址为 0x50 的 EEPROM 作为通信目标。接下来,我们使用 read 函数从 EEPROM 中读取 256 字节的数据,并使用 write 函数将 0 到 255 的数据写入 EEPROM 中。请注意,这是一个简单的示例,实际应用中需要根据 EEPROM 的型号和规格进行适当的修改。
i2c读写eeprom代码
I2C(Inter-Integrated Circuit)是用于连接微控制器和其他简单外围设备的一种串行通信协议。以下是使用Python的SMBus库(适用于Linux和macOS)读写EEPROM(如AT24XX系列)的基本示例代码:
```python
import smbus
# 初始化I2C总线
bus = smbus.SMBus(1) # 如果你在主板上只有一个I2C端口,通常选择1
# I2C地址,根据你的EEPROM型号替换
EEPROM_ADDRESS = 0x50
# 打开EEPROM设备
device = bus.read_i2c_block_data(EEPROM_ADDRESS, 0)
def read_eeprom(address):
byte_array = bus.read_byte_data(EEPROM_ADDRESS, address)
return byte_array
def write_eeprom(address, value):
bus.write_byte_data(EEPROM_ADDRESS, address, value)
# 示例读取某个位置的数据
data = read_eeprom(1) # 从地址1开始读取
print("Read data from address 1:", hex(data))
# 示例写入数据
write_eeprom(5, 0x42) # 将0x42写入地址5
print("Wrote 0x42 to address 5.")
# 关闭I2C
bus.close()
阅读全文
相关推荐
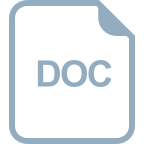
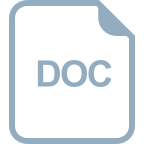
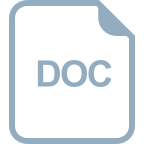

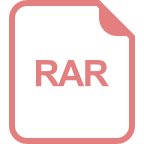
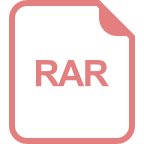
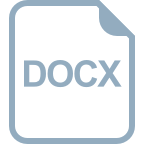
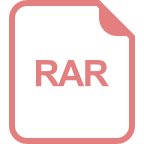
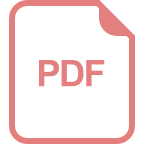
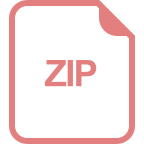
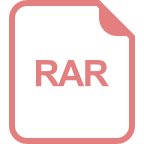
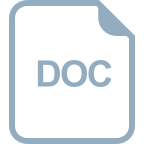
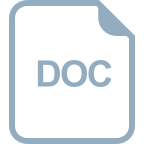
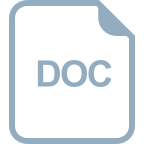
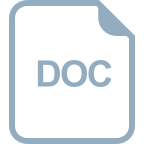
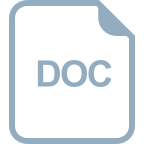