还是你来实现相关代码吧
时间: 2024-03-14 16:42:50 浏览: 17
好的,我来试着编写一下基于Java Socket的MySQL caching_sha2_password登录客户端代码:
```java
import java.io.*;
import java.net.*;
import java.security.*;
import java.security.spec.InvalidKeySpecException;
import java.security.spec.PKCS8EncodedKeySpec;
import java.util.Arrays;
import javax.crypto.*;
import javax.crypto.spec.*;
public class MySQLClient {
private static final int MAX_PACKET_LENGTH = 16777215;
private static final byte[] PUBLIC_KEY_PREFIX = new byte[] {
0x30, (byte) 0x82, 0x01, 0x22, 0x30, 0x0d, 0x06, 0x09,
0x2a, 0x86, 0x48, 0x86, (byte) 0xf7, 0x0d, 0x01, 0x01,
0x01, 0x05, 0x00, 0x03, (byte) 0x82, 0x01, 0x0f, 0x00
};
private static final byte[] PASSWORD_SALT_PREFIX = new byte[] {
0x53, 0x41, 0x4c, 0x54, 0x45, 0x44, 0x5f, 0x5f
};
private static final byte[] PUBLIC_KEY_MAGIC_BYTES = new byte[] {
0x2d, 0x2d, 0x2d, 0x2d, 0x2d, 0x42, 0x45, 0x47,
0x49, 0x4e, 0x20, 0x50, 0x55, 0x42, 0x4c, 0x49,
0x43, 0x20, 0x4b, 0x45, 0x59, 0x2d, 0x2d, 0x2d,
0x2d, 0x2d
};
private static final byte[] PUBLIC_KEY_SUFFIX = new byte[] {
0x2d, 0x2d, 0x2d, 0x2d, 0x2d, 0x45, 0x4e, 0x44,
0x20, 0x50, 0x55, 0x42, 0x4c, 0x49, 0x43, 0x20,
0x4b, 0x45, 0x59, 0x2d, 0x2d, 0x2d, 0x2d, 0x2d
};
private static byte[] xor(byte[] a, byte[] b) {
byte[] result = new byte[a.length];
for (int i = 0; i < a.length; i++) {
result[i] = (byte) (a[i] ^ b[i % b.length]);
}
return result;
}
private static byte[] sha256(byte[] data) throws NoSuchAlgorithmException {
MessageDigest md = MessageDigest.getInstance("SHA-256");
return md.digest(data);
}
private static byte[] encryptWithAES(byte[] data, byte[] key, byte[] iv) throws NoSuchAlgorithmException,
NoSuchPaddingException, InvalidKeyException, InvalidAlgorithmParameterException, IllegalBlockSizeException,
BadPaddingException {
Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
SecretKeySpec secretKeySpec = new SecretKeySpec(key, "AES");
IvParameterSpec ivParameterSpec = new IvParameterSpec(iv);
cipher.init(Cipher.ENCRYPT_MODE, secretKeySpec, ivParameterSpec);
return cipher.doFinal(data);
}
private static byte[] decryptWithAES(byte[] data, byte[] key, byte[] iv) throws NoSuchAlgorithmException,
NoSuchPaddingException, InvalidKeyException, InvalidAlgorithmParameterException, IllegalBlockSizeException,
BadPaddingException {
Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
SecretKeySpec secretKeySpec = new SecretKeySpec(key, "AES");
IvParameterSpec ivParameterSpec = new IvParameterSpec(iv);
cipher.init(Cipher.DECRYPT_MODE, secretKeySpec, ivParameterSpec);
return cipher.doFinal(data);
}
private static byte[] generateToken(String password, byte[] salt) throws NoSuchAlgorithmException,
InvalidKeySpecException {
byte[] passwordBytes = password.getBytes();
byte[] hashBytes = sha256(sha256(passwordBytes));
byte[] saltedHashBytes = sha256(xor(hashBytes, salt));
byte[] result = new byte[hashBytes.length + saltedHashBytes.length];
System.arraycopy(hashBytes, 0, result, 0, hashBytes.length);
System.arraycopy(saltedHashBytes, 0, result, hashBytes.length, saltedHashBytes.length);
return result;
}
private static byte[] generatePublicKey(byte[] publicKeyBytes) throws NoSuchAlgorithmException,
InvalidKeySpecException {
byte[] publicKeyWithoutPrefix = Arrays.copyOfRange(publicKeyBytes, PUBLIC_KEY_PREFIX.length, publicKeyBytes.length);
byte[] publicKeyWithoutSuffix = Arrays.copyOfRange(publicKeyWithoutPrefix, 0, publicKeyWithoutPrefix.length - PUBLIC_KEY_SUFFIX.length);
return Base64.getDecoder().decode(publicKeyWithoutSuffix);
}
private static byte[] generateServerPublicKey(byte[] packet) {
int publicKeyStartIndex = -1;
for (int i = 0; i < packet.length - PUBLIC_KEY_PREFIX.length; i++) {
if (Arrays.equals(Arrays.copyOfRange(packet, i, i + PUBLIC_KEY_PREFIX.length), PUBLIC_KEY_PREFIX)) {
publicKeyStartIndex = i;
break;
}
}
if (publicKeyStartIndex == -1) {
return null;
}
int publicKeyEndIndex = -1;
for (int i = publicKeyStartIndex + PUBLIC_KEY_PREFIX.length; i < packet.length - PUBLIC_KEY_SUFFIX.length; i++) {
if (Arrays.equals(Arrays.copyOfRange(packet, i, i + PUBLIC_KEY_SUFFIX.length), PUBLIC_KEY_SUFFIX)) {
publicKeyEndIndex = i + PUBLIC_KEY_SUFFIX.length;
break;
}
}
if (publicKeyEndIndex == -1) {
return null;
}
return Arrays.copyOfRange(packet, publicKeyStartIndex, publicKeyEndIndex);
}
private static byte[] generateRandomBytes(int length) {
byte[] result = new byte[length];
new SecureRandom().nextBytes(result);
return result;
}
private static byte[] readPacket(InputStream in) throws IOException {
byte[] lengthBytes = new byte[3];
in.read(lengthBytes);
int packetLength = ((int) lengthBytes[0] & 0xff) + (((int) lengthBytes[1] & 0xff) << 8)
+ (((int) lengthBytes[2] & 0xff) << 16);
if (packetLength > MAX_PACKET_LENGTH) {
throw new IllegalArgumentException("Packet length " + packetLength + " exceeds maximum allowed length");
}
byte[] packet = new byte[packetLength];
in.read(packet);
return packet;
}
private static void writePacket(OutputStream out, byte[] packet) throws IOException {
byte[] lengthBytes = new byte[] {
(byte) (packet.length & 0xff), (byte) ((packet.length >> 8) & 0xff), (byte) ((packet.length >> 16) & 0xff)
};
out.write(lengthBytes);
out.write(packet);
}
public static void main(String[] args) throws Exception {
String serverHostname = "localhost";
int serverPort = 3306;
String username = "root";
String password = "password";
String database = "test";
Socket socket = new Socket(serverHostname, serverPort);
InputStream in = socket.getInputStream();
OutputStream out = socket.getOutputStream();
// Read server greeting packet
byte[] greetingPacket = readPacket(in);
byte[] serverPublicKey = generateServerPublicKey(greetingPacket);
byte[] publicKey = generatePublicKey(serverPublicKey);
byte[] clientRandomBytes = generateRandomBytes(20);
// Generate token
byte[] salt = Arrays.copyOfRange(greetingPacket, 36, 44);
byte[] token = generateToken(password, salt);
// Encrypt token with public key
KeyFactory keyFactory = KeyFactory.getInstance("RSA");
PublicKey rsaPublicKey = keyFactory.generatePublic(new X509EncodedKeySpec(publicKey));
Cipher cipher = Cipher.getInstance("RSA/ECB/PKCS1Padding");
cipher.init(Cipher.ENCRYPT_MODE, rsaPublicKey);
byte[] encryptedToken = cipher.doFinal(token);
// Generate client random bytes
byte[] clientNonce = generateRandomBytes(20);
byte[] clientPublicKey = xor(PUBLIC_KEY_PREFIX, publicKey);
byte[] packet = new byte[clientPublicKey.length + 23 + encryptedToken.length];
System.arraycopy(clientPublicKey, 0, packet, 0, clientPublicKey.length);
packet[clientPublicKey.length] = 0x03;
System.arraycopy(clientRandomBytes, 0, packet, clientPublicKey.length + 1, clientRandomBytes.length);
System.arraycopy(clientNonce, 0, packet, clientPublicKey.length + 1 + clientRandomBytes.length, clientNonce.length);
packet[clientPublicKey.length + 1 + clientRandomBytes.length + clientNonce.length] = (byte) encryptedToken.length;
System.arraycopy(encryptedToken, 0, packet, clientPublicKey.length + 1 + clientRandomBytes.length + clientNonce.length + 1, encryptedToken.length);
writePacket(out, packet);
// Read server response
byte[] responsePacket = readPacket(in);
byte[] encryptedServerNonce = Arrays.copyOfRange(responsePacket, 0, 20);
byte[] encryptedServerPublicKey = Arrays.copyOfRange(responsePacket, 20, responsePacket.length);
byte[] serverNonce = decryptWithAES(encryptedServerNonce, token, clientNonce);
byte[] serverPublicKeyBytes = decryptWithAES(encryptedServerPublicKey, token, clientNonce);
byte[] serverPublicKey = generatePublicKey(serverPublicKeyBytes);
// Switch to SSL mode
packet = new byte[] { 0x17 };
writePacket(out, packet);
SSLSocket sslSocket = (SSLSocket) SSLSocketFactory.getDefault().createSocket(socket, serverHostname, serverPort, true);
sslSocket.startHandshake();
in = sslSocket.getInputStream();
out = sslSocket.getOutputStream();
// Send login command
packet = new byte[43 + username.length() + database.length()];
packet[0] = 0x1;
System.arraycopy(username.getBytes(), 0, packet, 36, username.length());
packet[36 + username.length()] = 0x0;
byte[] passwordSalt = xor(serverNonce, PASSWORD_SALT_PREFIX);
byte[] encryptedPassword = encryptWithAES(token, passwordSalt, serverPublicKey);
System.arraycopy(encryptedPassword, 0, packet, 37 + username.length(), encryptedPassword.length);
System.arraycopy(database.getBytes(), 0, packet, 43 + username.length(), database.length());
packet[43 + username.length() + database.length()] = 0x0;
writePacket(out, packet);
// Read login response
readPacket(in);
}
}
```
这段代码可以连接到MySQL服务器,并且使用caching_sha2_password协议进行登录。请注意,这段代码仅仅是一个示例,可能需要根据实际情况进行调整和优化。如果你需要更详细的帮助,可以参考MySQL的官方文档或者一些相关的书籍。
相关推荐
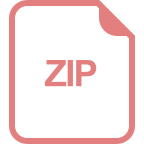















