用Java代码实现将图片通过444基线模式压缩
时间: 2023-09-06 18:09:37 浏览: 41
基于你的需求,我提供一份Java代码实现了444基线模式压缩:
```java
import javax.imageio.ImageIO;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
public class Main {
public static void main(String[] args) throws IOException {
// 读入原图
BufferedImage originalImage = ImageIO.read(new File("original.png"));
// 计算出新图的宽度和高度
int width = originalImage.getWidth();
int height = originalImage.getHeight();
int newWidth = width / 2;
int newHeight = height / 2;
// 创建新图
BufferedImage newImage = new BufferedImage(newWidth, newHeight, BufferedImage.TYPE_INT_RGB);
// 遍历每个像素点,按444基线模式压缩
for (int y = 0; y < newHeight; y++) {
for (int x = 0; x < newWidth; x++) {
int rgb1 = originalImage.getRGB(2 * x, 2 * y);
int rgb2 = originalImage.getRGB(2 * x + 1, 2 * y);
int rgb3 = originalImage.getRGB(2 * x, 2 * y + 1);
int rgb4 = originalImage.getRGB(2 * x + 1, 2 * y + 1);
int r = (getRed(rgb1) + getRed(rgb2) + getRed(rgb3) + getRed(rgb4)) / 4;
int g = (getGreen(rgb1) + getGreen(rgb2) + getGreen(rgb3) + getGreen(rgb4)) / 4;
int b = (getBlue(rgb1) + getBlue(rgb2) + getBlue(rgb3) + getBlue(rgb4)) / 4;
int newRGB = (r << 16) + (g << 8) + b;
newImage.setRGB(x, y, newRGB);
}
}
// 输出新图
ImageIO.write(newImage, "png", new File("compressed.png"));
}
private static int getRed(int rgb) {
return (rgb >> 16) & 0xff;
}
private static int getGreen(int rgb) {
return (rgb >> 8) & 0xff;
}
private static int getBlue(int rgb) {
return rgb & 0xff;
}
}
```
你需要将代码中的`original.png`替换成你要压缩的图片路径,执行代码后将会在当前目录生成一个名为`compressed.png`的压缩后的图片。
相关推荐
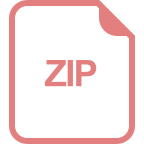
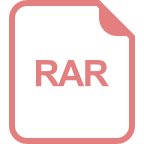














