【Java图形算法优化】:数据压缩与算法效率提升指南
发布时间: 2024-08-29 16:50:33 阅读量: 46 订阅数: 42 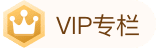
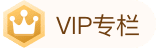
# 1. Java图形算法优化概述
在当今这个信息时代,图形算法的应用无处不在,从简单的用户界面元素到复杂的三维动画和游戏渲染,它们都在背后默默地提供支持。对于Java开发者而言,理解和优化图形算法是提升应用性能和用户体验的关键。本章将对Java图形算法优化进行概述,探讨它的重要性以及在开发过程中可能会遇到的挑战和解决方案。
优化图形算法不仅仅是提高效率,它还包括改善用户体验,减少应用程序的内存占用,以及降低计算资源的消耗。随着移动设备和嵌入式系统的普及,图形算法优化在资源受限的环境中显得尤为重要。本章将从理论与实践两个维度出发,为读者提供一个全面的图形算法优化视角。
# 2. 数据压缩基础与技术
### 2.1 数据压缩的理论基础
#### 2.1.1 压缩的必要性与优势
在当今的信息时代,数据量呈指数级增长,这对数据存储与传输提出了更高的要求。数据压缩技术应运而生,它通过特定的算法减少数据的大小,有效节省存储空间和传输带宽,同时还能缩短数据处理时间。压缩的优势不仅仅体现在减少存储成本上,还可以提高数据处理速度,降低能耗,从而在众多IT系统中扮演了关键角色。
#### 2.1.2 常见的数据压缩算法
数据压缩可以分为无损压缩和有损压缩两类。无损压缩算法在压缩和解压过程中保证数据完全一致,常见的无损压缩算法包括霍夫曼编码、Lempel-Ziv编码和算术编码。有损压缩则允许在压缩过程中损失部分数据,以获得更高的压缩比,例如JPEG和MP3格式文件的压缩就属于有损压缩。
### 2.2 压缩技术的实践应用
#### 2.2.1 字符编码压缩
字符编码压缩主要应用于文本数据。以霍夫曼编码为例,它是一种变长编码方法,将出现频率高的字符用较短的编码表示,频率低的字符则用较长的编码。这种方法有效减少了文本文件的大小,提高了存储效率。
```java
import java.util.PriorityQueue;
import java.util.HashMap;
import java.util.Map;
public class HuffmanCoding {
public Map<Character, String> compress(String text) {
Map<Character, Integer> freqMap = new HashMap<>();
for (char c : text.toCharArray()) {
freqMap.put(c, freqMap.getOrDefault(c, 0) + 1);
}
PriorityQueue<HuffmanNode> pq = new PriorityQueue<>();
for (Map.Entry<Character, Integer> entry : freqMap.entrySet()) {
pq.add(new HuffmanNode(entry.getKey(), entry.getValue()));
}
while (pq.size() > 1) {
HuffmanNode left = pq.poll();
HuffmanNode right = pq.poll();
HuffmanNode sum = new HuffmanNode('\0', left.frequency + right.frequency);
sum.left = left;
sum.right = right;
pq.add(sum);
}
Map<Character, String> codes = new HashMap<>();
buildCode(pq.poll(), "", codes);
return codes;
}
private void buildCode(HuffmanNode root, String str, Map<Character, String> codes) {
if (root.left == null && root.right == null) {
codes.put(root.c, str);
return;
}
buildCode(root.left, str + "0", codes);
buildCode(root.right, str + "1", codes);
}
private class HuffmanNode implements Comparable<HuffmanNode> {
char c;
int frequency;
HuffmanNode left;
HuffmanNode right;
HuffmanNode(char c, int frequency) {
this.c = c;
this.frequency = frequency;
}
@Override
public int compareTo(HuffmanNode that) {
return this.frequency - that.frequency;
}
}
}
```
上述代码展示了如何构建一个霍夫曼树,并为每个字符生成编码。
#### 2.2.2 图像与视频压缩方法
图像和视频压缩方法如JPEG和H.264都是经过精心设计的,它们利用了图像数据的空间相关性和时间冗余性。例如,JPEG压缩使用了离散余弦变换(DCT),将图像从空间域转换到频率域,然后对高频系数进行量化和编码,丢弃人眼不易察觉的细节,从而实现压缩。
#### 2.2.3 实际案例分析
一个实际案例是使用LZW算法(Lempel-Ziv-Welch)在GIF图像格式中进行压缩。LZW算法是一种字典编码方法,通过维护一个字符串字典来存储重复出现的字符串序列,然后使用较短的代码替换,以达到压缩目的。
### 2.3 压缩算法的效率评估
#### 2.3.1 时间复杂度和空间复杂度分析
评估压缩算法的效率,需要分析算法的时间复杂度和空间复杂度。时间复杂度反映了算法处理数据所需的时间随着数据量增加的变化趋势,空间复杂度则反映了算法运行过程中占用的空间资源。一个好的压缩算法应该具有较低的时间复杂度和空间复杂度。
#### 2.3.2 性能测试与结果解读
性能测试可以通过构建测试集,使用压缩算法对数据进行压缩,然后记录压缩和解压过程所需的时间,以及压缩后的数据大小。通过对不同算法的性能测试结果进行对比,可以得出各算法的适用场景和优势。
## 第三章:Java图形处理中的算法优化
### 3.1 图形渲染性能提升技巧
#### 3.1.1 渲染管线的优化
图形渲染管线是处理图形数据到最终显示在屏幕上的过程。优化渲染管线可以大幅度提升图形渲染效率。比如,合理使用批处理和异步加载可以减少图形引擎的工作量。另外,利用现代GPU的并行处理能力,可以进一步优化渲染速度。
#### 3.1.2 硬件加速技术的应用
硬件加速技术通过利用GPU进行图形计算,减少了CPU的负担。在Java中,可以利用JavaFX或OpenGL等图形库来实现硬件加速。通过使用这些库的专用API,可以将复杂的图形渲染任务委托给GPU执行,从而提高渲染效率。
### 3.2 Java图形算法的改进方法
#### 3.2.1 算法分解与并行处理
在处理复杂图形算法时
0
0
相关推荐
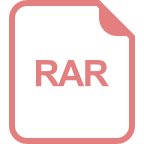
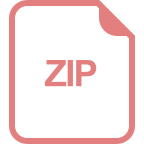
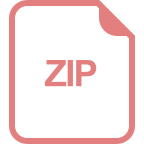
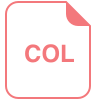
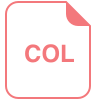
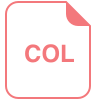

