用javaweb写一个简单的通知公告网页代码
时间: 2023-10-06 10:12:26 浏览: 271
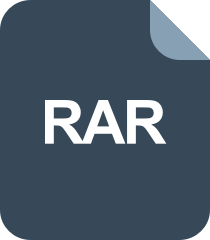
java 写的一个消息发布网站源码
好的,下面是一个简单的通知公告网页代码示例:
1.公告列表页面notice_list.jsp:
```html
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>通知公告</title>
<link rel="stylesheet" href="https://cdn.bootcss.com/bootstrap/3.3.7/css/bootstrap.min.css">
<script src="https://cdn.bootcss.com/jquery/3.2.1/jquery.min.js"></script>
<script src="https://cdn.bootcss.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
</head>
<body>
<div class="container">
<h1>通知公告</h1>
<table class="table table-striped">
<thead>
<tr>
<th>标题</th>
<th>创建时间</th>
</tr>
</thead>
<tbody>
<c:forEach items="${notices}" var="notice">
<tr>
<td><a href="notice_detail.jsp?id=${notice.id}">${notice.title}</a></td>
<td>${notice.createTime}</td>
</tr>
</c:forEach>
</tbody>
</table>
<a href="notice_add.jsp" class="btn btn-primary">添加公告</a>
</div>
</body>
</html>
```
2.公告详情页面notice_detail.jsp:
```html
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>${notice.title}</title>
<link rel="stylesheet" href="https://cdn.bootcss.com/bootstrap/3.3.7/css/bootstrap.min.css">
<script src="https://cdn.bootcss.com/jquery/3.2.1/jquery.min.js"></script>
<script src="https://cdn.bootcss.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
</head>
<body>
<div class="container">
<h1>${notice.title}</h1>
<p>${notice.content}</p>
<p>${notice.createTime}</p>
<a href="notice_list.jsp" class="btn btn-default">返回列表</a>
</div>
</body>
</html>
```
3.添加公告页面notice_add.jsp:
```html
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>添加公告</title>
<link rel="stylesheet" href="https://cdn.bootcss.com/bootstrap/3.3.7/css/bootstrap.min.css">
<script src="https://cdn.bootcss.com/jquery/3.2.1/jquery.min.js"></script>
<script src="https://cdn.bootcss.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
</head>
<body>
<div class="container">
<h1>添加公告</h1>
<form role="form" action="notice_add.do" method="post">
<div class="form-group">
<label for="title">标题</label>
<input type="text" class="form-control" id="title" name="title">
</div>
<div class="form-group">
<label for="content">内容</label>
<textarea class="form-control" id="content" name="content"></textarea>
</div>
<button type="submit" class="btn btn-primary">提交</button>
<a href="notice_list.jsp" class="btn btn-default">取消</a>
</form>
</div>
</body>
</html>
```
4.公告列表页面对应的Servlet代码NoticeListServlet.java:
```java
package com.example.servlet;
import java.io.IOException;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.example.dao.NoticeDao;
import com.example.model.Notice;
@WebServlet("/notice_list.jsp")
public class NoticeListServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private NoticeDao noticeDao;
public NoticeListServlet() {
super();
noticeDao = new NoticeDao();
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
List<Notice> notices = noticeDao.getAll();
request.setAttribute("notices", notices);
request.getRequestDispatcher("/WEB-INF/jsp/notice_list.jsp").forward(request, response);
}
}
```
5.公告详情页面对应的Servlet代码NoticeDetailServlet.java:
```java
package com.example.servlet;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.example.dao.NoticeDao;
import com.example.model.Notice;
@WebServlet("/notice_detail.jsp")
public class NoticeDetailServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private NoticeDao noticeDao;
public NoticeDetailServlet() {
super();
noticeDao = new NoticeDao();
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
int id = Integer.parseInt(request.getParameter("id"));
Notice notice = noticeDao.getById(id);
request.setAttribute("notice", notice);
request.getRequestDispatcher("/WEB-INF/jsp/notice_detail.jsp").forward(request, response);
}
}
```
6.添加公告页面对应的Servlet代码NoticeAddServlet.java:
```java
package com.example.servlet;
import java.io.IOException;
import java.time.LocalDateTime;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.example.dao.NoticeDao;
import com.example.model.Notice;
@WebServlet("/notice_add.do")
public class NoticeAddServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private NoticeDao noticeDao;
public NoticeAddServlet() {
super();
noticeDao = new NoticeDao();
}
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String title = request.getParameter("title");
String content = request.getParameter("content");
LocalDateTime createTime = LocalDateTime.now();
Notice notice = new Notice(title, content, createTime);
noticeDao.add(notice);
response.sendRedirect("notice_list.jsp");
}
}
```
以上代码仅供参考,具体实现可以根据具体需求进行调整。
阅读全文
相关推荐

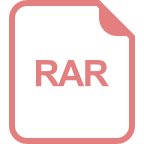
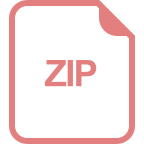
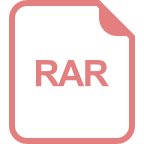
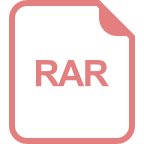
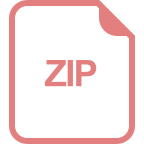
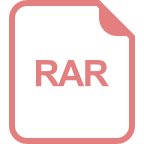
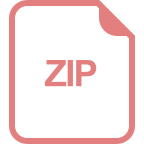
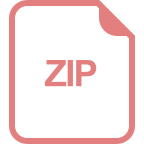
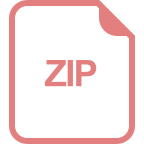
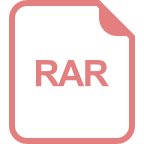
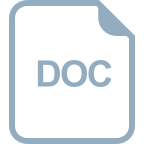
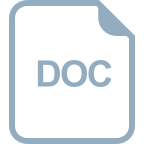
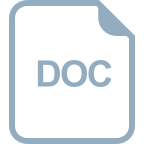
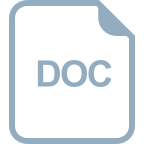
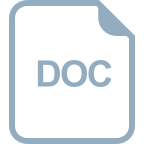