用Javaweb写一个购物车的代码
时间: 2023-05-11 21:07:18 浏览: 136
好的,以下是一个简单的Javaweb购物车代码:
1. 创建一个名为ShoppingCart的Java类:
public class ShoppingCart {
private Map<String, Integer> items = new HashMap<String, Integer>();
public void addItem(String itemId, int quantity) {
if (items.containsKey(itemId)) {
int currentQuantity = items.get(itemId);
items.put(itemId, currentQuantity + quantity);
} else {
items.put(itemId, quantity);
}
}
public void removeItem(String itemId) {
items.remove(itemId);
}
public Map<String, Integer> getItems() {
return items;
}
public int getTotalItems() {
int totalItems = 0;
for (int quantity : items.values()) {
totalItems += quantity;
}
return totalItems;
}
public double getTotalPrice() {
double totalPrice = 0.0;
for (Map.Entry<String, Integer> entry : items.entrySet()) {
String itemId = entry.getKey();
int quantity = entry.getValue();
double price = getItemPrice(itemId);
totalPrice += price * quantity;
}
return totalPrice;
}
private double getItemPrice(String itemId) {
// TODO: 根据商品ID获取商品价格
return 0.0;
}
}
2. 在JSP页面中使用购物车:
<%@ page import="com.example.ShoppingCart" %>
<%
ShoppingCart cart = (ShoppingCart) session.getAttribute("cart");
if (cart == null) {
cart = new ShoppingCart();
session.setAttribute("cart", cart);
}
%>
<!-- 在页面中展示购物车信息 -->
<p>购物车中有 <%= cart.getTotalItems() %> 件商品,总价值为 <%= cart.getTotalPrice() %> 元。</p>
<!-- 添加商品到购物车 -->
<%
String itemId = request.getParameter("itemId");
int quantity = Integer.parseInt(request.getParameter("quantity"));
cart.addItem(itemId, quantity);
%>
<!-- 从购物车中删除商品 -->
<%
String itemId = request.getParameter("itemId");
cart.removeItem(itemId);
%>
注意:这只是一个简单的示例代码,实际上购物车的实现可能更加复杂,需要考虑并发访问、商品库存等问题。
相关推荐
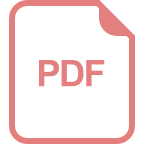
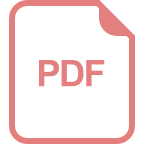
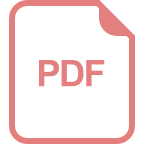
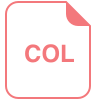
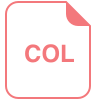
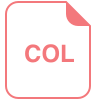
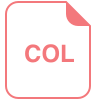
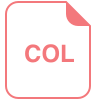









