javaweb实现购物车功能代码
时间: 2023-11-10 09:05:08 浏览: 135
好的,以下是一个简单的JavaWeb购物车功能代码示例:
首先,我们需要创建一个Cart类来表示购物车:
```java
public class Cart {
private Map<String, Integer> items = new HashMap<>();
public void addItem(String itemId, int quantity) {
if (items.containsKey(itemId)) {
int oldQuantity = items.get(itemId);
items.put(itemId, oldQuantity + quantity);
} else {
items.put(itemId, quantity);
}
}
public void removeItem(String itemId) {
items.remove(itemId);
}
public Map<String, Integer> getItems() {
return items;
}
public int getItemCount() {
int count = 0;
for (int quantity : items.values()) {
count += quantity;
}
return count;
}
public double getTotalPrice() {
double totalPrice = 0;
for (Map.Entry<String, Integer> entry : items.entrySet()) {
String itemId = entry.getKey();
int quantity = entry.getValue();
double itemPrice = getItemPrice(itemId);
totalPrice += itemPrice * quantity;
}
return totalPrice;
}
private double getItemPrice(String itemId) {
// TODO: 根据 itemId 查询商品价格,这里省略实现
return 0;
}
}
```
以上代码中,我们使用了一个HashMap来存储购物车中的商品信息。addItem()方法用于向购物车中添加商品,removeItem()方法用于从购物车中移除商品,getItems()方法用于获取购物车中所有商品信息,getItemCount()方法用于获取购物车中商品数量,getTotalPrice()方法用于获取购物车中商品总价,getItemPrice()方法用于根据商品ID查询商品价格。
接下来,我们需要编写一个Servlet来处理用户的购物车请求:
```java
public class CartServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String action = request.getParameter("action");
HttpSession session = request.getSession();
Cart cart = (Cart) session.getAttribute("cart");
if (cart == null) {
cart = new Cart();
session.setAttribute("cart", cart);
}
if (action.equals("add")) {
String itemId = request.getParameter("itemId");
int quantity = Integer.parseInt(request.getParameter("quantity"));
cart.addItem(itemId, quantity);
response.sendRedirect(request.getContextPath() + "/cart.jsp");
} else if (action.equals("remove")) {
String itemId = request.getParameter("itemId");
cart.removeItem(itemId);
response.sendRedirect(request.getContextPath() + "/cart.jsp");
}
}
}
```
以上代码中,我们首先获取用户的请求操作类型(添加商品或移除商品),然后从Session中获取购物车对象,如果购物车对象不存在,则创建一个新的购物车对象并存储到Session中。
如果用户请求添加商品,则获取商品ID和数量,然后调用购物车对象的addItem()方法添加商品并重定向到购物车页面;如果用户请求移除商品,则获取商品ID,然后调用购物车对象的removeItem()方法移除商品并重定向到购物车页面。
最后,我们需要在购物车页面中显示购物车信息:
```html
<%
Cart cart = (Cart) session.getAttribute("cart");
%>
<table>
<thead>
<tr>
<th>商品ID</th>
<th>商品名称</th>
<th>数量</th>
<th>价格</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<% for (Map.Entry<String, Integer> entry : cart.getItems().entrySet()) { %>
<tr>
<td><%= entry.getKey() %></td>
<td><%= getItemName(entry.getKey()) %></td>
<td><%= entry.getValue() %></td>
<td><%= getItemPrice(entry.getKey()) %></td>
<td>
<form method="post" action="<%= request.getContextPath() %>/cart">
<input type="hidden" name="action" value="remove">
<input type="hidden" name="itemId" value="<%= entry.getKey() %>">
<button type="submit">移除</button>
</form>
</td>
</tr>
<% } %>
</tbody>
</table>
<p>总计:¥ <%= cart.getTotalPrice() %></p>
```
以上代码中,我们首先从Session中获取购物车对象,然后使用一个表格来显示购物车中的所有商品信息。对于每个商品,我们显示商品ID、商品名称、数量、价格和一个移除按钮。当用户点击移除按钮时,会向Servlet发送一个POST请求,并将操作类型和商品ID作为参数传递过去。最后,我们还显示了购物车中所有商品的总价。
以上就是一个简单的JavaWeb购物车功能代码示例,仅供参考。实际项目中可能会更加复杂,需要根据具体需求进行调整和优化。
阅读全文
相关推荐
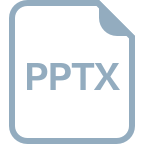
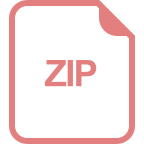




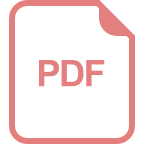
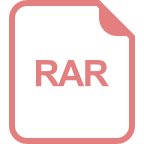
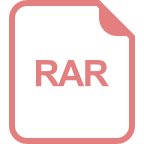
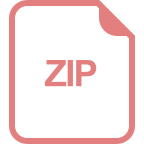





