java8写一个从配置文件中获取多个条件,并将list中满足条件的数据skip掉的共通方法
时间: 2024-05-02 14:21:42 浏览: 75
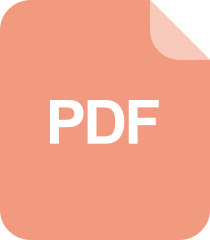
java8新特性将List中按指定属性排序过滤重复数据的方法

下面是一个示例代码,可以从配置文件中读取多个条件,并在一个List中跳过满足条件的元素:
```java
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.List;
import java.util.Properties;
import java.util.function.Predicate;
public class ConfigSkipper {
public static <T> void skipFromConfig(List<T> list, String configFile) {
Properties props = loadProperties(configFile);
for (String propName : props.stringPropertyNames()) {
String propValue = props.getProperty(propName);
Predicate<T> predicate = createPredicate(propName, propValue);
list.removeIf(predicate);
}
}
private static Properties loadProperties(String configFile) {
Properties props = new Properties();
try (InputStream is = new FileInputStream(configFile)) {
props.load(is);
} catch (IOException e) {
throw new RuntimeException("Failed to load configuration file: " + configFile, e);
}
return props;
}
private static <T> Predicate<T> createPredicate(String propName, String propValue) {
return new Predicate<T>() {
@Override
public boolean test(T t) {
try {
Object value = t.getClass().getField(propName).get(t);
return value.equals(propValue);
} catch (NoSuchFieldException | IllegalAccessException e) {
throw new RuntimeException("Failed to access field " + propName + " of object " + t, e);
}
}
};
}
}
```
这个方法的思路如下:
1. 从配置文件中读取所有的条件;
2. 对于每个条件,创建一个Predicate对象,这个Predicate对象检查对象中的指定属性是否等于指定值;
3. 使用List的removeIf方法,将满足条件的元素从列表中移除。
注意,这个方法要求对象中的属性名与配置文件中的条件名相同,并且这些属性必须是公有的。如果不符合这些条件,可能会导致运行时异常。如果要更通用地处理不同类型的对象和属性,可以考虑使用反射来实现。
阅读全文
相关推荐
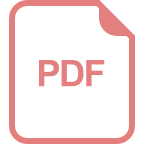
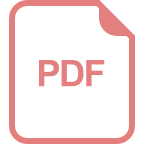




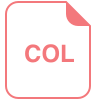
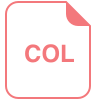
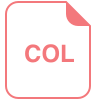
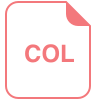
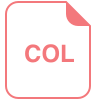
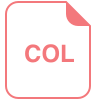
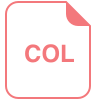
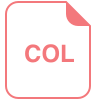
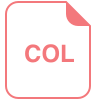
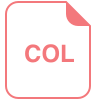
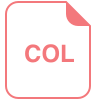