java8写一个通过配置文件中的多重条件,对list中满足全部条件的数据进行skip处理的共通方法
时间: 2024-05-06 18:22:04 浏览: 17
假设我们的配置文件是一个properties文件,里面有多个条件,格式如下:
```
condition1=value1
condition2=value2
condition3=value3
```
我们可以通过以下方法将这些条件读取到一个Map中:
```java
public static Map<String, String> readConfig(String filePath) {
Map<String, String> configMap = new HashMap<>();
Properties props = new Properties();
try (InputStream input = new FileInputStream(filePath)) {
props.load(input);
for (String key : props.stringPropertyNames()) {
configMap.put(key, props.getProperty(key));
}
} catch (IOException ex) {
ex.printStackTrace();
}
return configMap;
}
```
接下来,我们可以定义一个通用方法,通过传入一个List和一个配置Map,对List中满足全部条件的数据进行skip处理:
```java
public static <T> List<T> skipByConditions(List<T> list, Map<String, String> configMap) {
return list.stream()
.filter(item -> {
for (String key : configMap.keySet()) {
if (!configMap.get(key).equals(item.getProperty(key))) {
return false;
}
}
return true;
})
.skip(configMap.size())
.collect(Collectors.toList());
}
```
在这个方法中,我们首先使用stream()方法将List转换成一个Stream。然后,我们使用filter()方法对Stream中的元素进行筛选。我们遍历配置Map中的每一个条件,如果当前元素的属性值与配置值不相等,则说明该元素不符合条件,返回false。如果当前元素符合所有条件,则返回true。最后,我们使用skip()方法跳过满足条件的前n个元素,其中n为配置Map中条件的数量。最后,我们使用collect()方法将Stream转换回List。
相关推荐
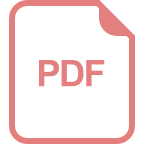














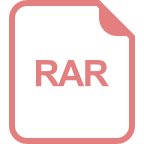