博弈树搜索__五子棋Python代码
时间: 2023-12-25 19:20:50 浏览: 120
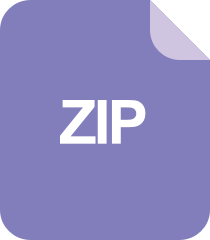
python五子棋代码
以下是五子棋的Python代码,使用了博弈树搜索算法。
```python
import copy
# 棋盘大小
BOARD_SIZE = 15
# 空白点
BLANK = 0
# 黑子
BLACK = 1
# 白子
WHITE = 2
class Board:
def __init__(self):
# 初始化棋盘
self.board = [[BLANK] * BOARD_SIZE for _ in range(BOARD_SIZE)]
# 初始化当前下棋方
self.current_player = BLACK
def get_current_player(self):
return self.current_player
def set_current_player(self, player):
self.current_player = player
def get_board(self):
return self.board
def set_board(self, board):
self.board = board
def get_blank_positions(self):
# 获取空白点位置
positions = []
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if self.board[i][j] == BLANK:
positions.append((i, j))
return positions
def is_valid_position(self, position):
# 判断位置是否在棋盘内
return 0 <= position[0] < BOARD_SIZE and 0 <= position[1] < BOARD_SIZE
def is_blank_position(self, position):
# 判断位置是否为空白点
return self.board[position[0]][position[1]] == BLANK
def is_win(self, player):
# 判断是否获胜
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if self.board[i][j] == player:
# 水平方向
if j <= BOARD_SIZE - 5 and self.board[i][j:j+5] == [player] * 5:
return True
# 垂直方向
if i <= BOARD_SIZE - 5 and [self.board[k][j] for k in range(i, i+5)] == [player] * 5:
return True
# 正斜方向
if i <= BOARD_SIZE - 5 and j <= BOARD_SIZE - 5 and [self.board[i+k][j+k] for k in range(5)] == [player] * 5:
return True
# 反斜方向
if i >= 4 and j <= BOARD_SIZE - 5 and [self.board[i-k][j+k] for k in range(5)] == [player] * 5:
return True
return False
class Game:
def __init__(self):
self.board = Board()
def get_available_positions(self):
# 获取可下棋位置
return self.board.get_blank_positions()
def play(self, position):
# 下棋
if self.board.is_valid_position(position) and self.board.is_blank_position(position):
self.board.get_board()[position[0]][position[1]] = self.board.get_current_player()
return True
return False
def switch_player(self):
# 切换下棋方
current_player = self.board.get_current_player()
if current_player == BLACK:
self.board.set_current_player(WHITE)
else:
self.board.set_current_player(BLACK)
def is_win(self):
# 判断是否获胜
return self.board.is_win(BLACK) or self.board.is_win(WHITE)
def is_draw(self):
# 判断是否平局
return len(self.get_available_positions()) == 0
class AI:
def __init__(self, game):
self.game = game
def get_move(self):
# 博弈树搜索算法
best_score = -float('inf')
best_move = None
for position in self.game.get_available_positions():
new_game = copy.deepcopy(self.game)
new_game.play(position)
score = self.minimax(new_game, 2, False)
if score > best_score:
best_score = score
best_move = position
return best_move
def minimax(self, game, depth, is_maximizing):
# 极小化极大算法
if depth == 0 or game.is_win() or game.is_draw():
return self.evaluate(game)
if is_maximizing:
best_score = -float('inf')
for position in game.get_available_positions():
new_game = copy.deepcopy(game)
new_game.play(position)
score = self.minimax(new_game, depth - 1, False)
best_score = max(best_score, score)
return best_score
else:
best_score = float('inf')
for position in game.get_available_positions():
new_game = copy.deepcopy(game)
new_game.play(position)
score = self.minimax(new_game, depth - 1, True)
best_score = min(best_score, score)
return best_score
def evaluate(self, game):
# 评估函数
if game.is_win():
if game.board.get_current_player() == BLACK:
return 1
else:
return -1
else:
return 0
def main():
game = Game()
ai = AI(game)
while True:
# 人类玩家下棋
print(game.board.get_board())
human_position = tuple(map(int, input("Your move (row, col): ").split(',')))
while not game.play(human_position):
print("Invalid move!")
human_position = tuple(map(int, input("Your move (row, col): ").split(',')))
if game.is_win():
print(game.board.get_board())
print("You win!")
break
if game.is_draw():
print(game.board.get_board())
print("Draw!")
break
# AI下棋
ai_position = ai.get_move()
print("AI's move:", ai_position)
game.play(ai_position)
if game.is_win():
print(game.board.get_board())
print("AI wins!")
break
if game.is_draw():
print(game.board.get_board())
print("Draw!")
break
if __name__ == '__main__':
main()
```
阅读全文
相关推荐
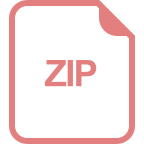
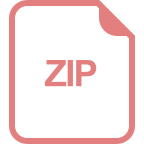
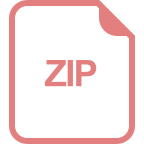
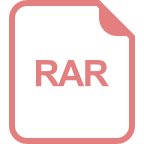
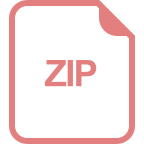
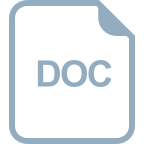
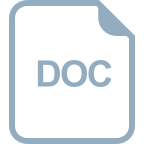



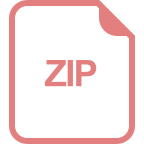
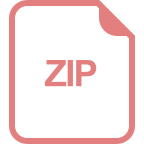
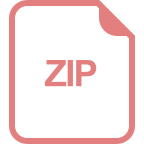
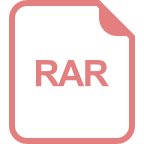
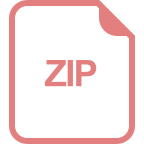
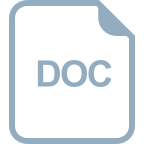

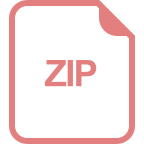