创建一个Javaweb在线考试系统源代码
时间: 2023-08-03 13:05:02 浏览: 46
很抱歉,我作为AI并不能提供完整的JavaWeb在线考试系统的源代码。不过,以下是一个简单的JavaWeb在线考试系统的实现思路和部分关键代码,可以供您参考:
1. 确定需求:在线考试系统需要支持考试科目、试题库、考试规则、考试记录等功能。
2. 设计数据库:根据需求设计数据库,包括考试科目表、试题库表、考试规则表、考试记录表等。
3. 编写后端代码:使用Java语言和Spring框架编写后端代码,包括数据访问层、业务逻辑层和控制层。
```java
// 数据访问层
@Repository
public interface ExamRepository extends JpaRepository<Exam, Long> {
}
// 业务逻辑层
@Service
public class ExamService {
@Autowired
private ExamRepository examRepository;
public List<Exam> getExams() {
return examRepository.findAll();
}
public Exam getExamById(Long id) {
return examRepository.findById(id).orElse(null);
}
}
// 控制层
@RestController
@RequestMapping("/api/exams")
public class ExamController {
@Autowired
private ExamService examService;
@GetMapping("")
public List<Exam> getExams() {
return examService.getExams();
}
@GetMapping("/{id}")
public Exam getExamById(@PathVariable Long id) {
return examService.getExamById(id);
}
}
```
4. 编写前端页面:使用HTML、CSS和JavaScript等技术编写前端页面,包括登录页面、考试页面、考试结果页面等。
```html
<!-- 登录页面 -->
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>登录</title>
</head>
<body>
<form action="/api/login" method="post">
<label for="username">用户名:</label>
<input type="text" id="username" name="username"><br>
<label for="password">密码:</label>
<input type="password" id="password" name="password"><br>
<button type="submit">登录</button>
</form>
</body>
</html>
<!-- 考试页面 -->
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>考试</title>
</head>
<body>
<h1>考试题目</h1>
<form action="/api/submit" method="post">
<label for="answer1">问题1:</label>
<input type="text" id="answer1" name="answer1"><br>
<label for="answer2">问题2:</label>
<input type="text" id="answer2" name="answer2"><br>
<button type="submit">提交</button>
</form>
</body>
</html>
<!-- 考试结果页面 -->
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>考试结果</title>
</head>
<body>
<h1>考试结果</h1>
<p>问题1答案:{{ answer1 }}</p>
<p>问题2答案:{{ answer2 }}</p>
<p>得分:{{ score }}</p>
</body>
</html>
```
以上是一个简单的JavaWeb在线考试系统的实现思路和部分关键代码,供您参考。请注意,这只是一个简单的示例,实际项目中可能需要更多的功能和代码实现。
相关推荐
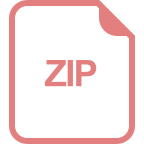
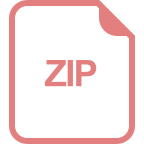














