c语言字符串替换
时间: 2023-06-22 17:29:44 浏览: 125
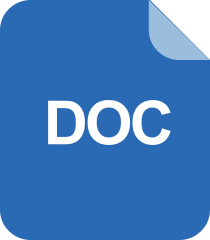
C语言字符串操作函数

在C语言中,可以使用`str_replace`函数实现字符串替换的功能。不过需要注意的是,C语言标准库中并没有提供`str_replace`函数,需要自己实现该函数或者使用第三方库。
以下是一个简单的`str_replace`函数实现:
```c
#include <stdio.h>
#include <string.h>
char * str_replace(char *str, const char *find, const char *replace)
{
char *temp = NULL;
char *p = NULL;
char *old = NULL;
size_t old_size = 0;
size_t replace_size = 0;
size_t len = 0;
size_t count = 0;
old_size = strlen(find);
replace_size = strlen(replace);
// 统计需要替换的次数
p = strstr(str, find);
while (p != NULL) {
count++;
p = strstr(p + old_size, find);
}
// 计算替换后的字符串总长度
len = strlen(str) + count * (replace_size - old_size);
// 分配新的字符串内存
temp = (char *)malloc(len + 1);
if (temp == NULL) {
return NULL;
}
// 开始替换
old = str;
p = strstr(str, find);
while (p != NULL) {
memcpy(temp + strlen(temp), old, p - old);
memcpy(temp + strlen(temp), replace, replace_size);
old = p + old_size;
p = strstr(old, find);
}
memcpy(temp + strlen(temp), old, strlen(str) - (old - str));
temp[len] = '\0';
return temp;
}
int main()
{
char str[] = "hello world, hello C";
char *new_str = NULL;
printf("original string: %s\n", str);
new_str = str_replace(str, "hello", "hi");
if (new_str != NULL) {
printf("new string: %s\n", new_str);
free(new_str);
}
return 0;
}
```
该函数会将原字符串中所有的`find`子串替换为`replace`子串,并返回新的字符串。在这个例子中,原字符串是"hello world, hello C",将其中的"hello"替换为"hi"后,新的字符串是"hi world, hi C"。
阅读全文
相关推荐
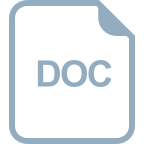
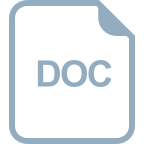

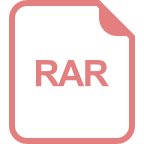
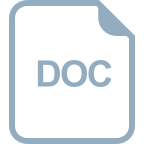
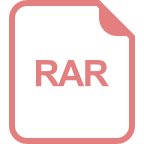
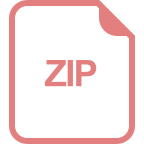
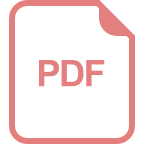
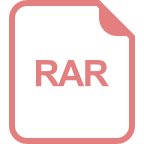
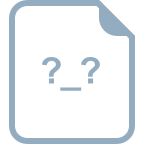
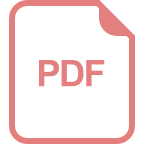
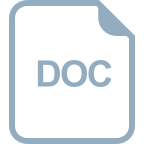
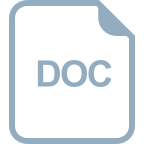
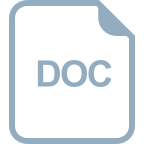
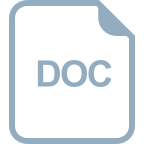
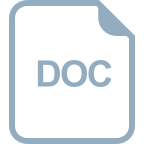
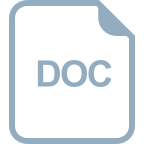