python 实现 LRUCache
时间: 2023-01-30 11:58:26 浏览: 83
当然可以! LRUCache 是一种常用的缓存策略,其中“LRU”指的是“最近最少使用”。它的工作原理是,如果缓存中已经存在所需要的数据,则直接返回该数据,否则从源头获取数据并加入到缓存中。为了使缓存保持有限的大小,当需要加入新数据时,缓存会把最近最少使用的数据清除出去,以确保缓存中只保留最常用的数据。
下面是一个用 Python 实现的 LRUCache 的简单例子:
```python
class LRUCache:
def __init__(self, capacity: int):
self.capacity = capacity
self.cache = {}
self.lru = []
def get(self, key: int) -> int:
if key not in self.cache:
return -1
self.lru.remove(key)
self.lru.append(key)
return self.cache[key]
def put(self, key: int, value: int) -> None:
if key in self.cache:
self.lru.remove(key)
elif len(self.cache) >= self.capacity:
del self.cache[self.lru[0]]
self.lru.pop(0)
self.cache[key] = value
self.lru.append(key)
```
其中,`self.cache` 用于存储缓存的数据,`self.lru` 用于记录数据的使用情况,用于找到最近最少使用的数据。
注意:这只是一个简单的例子,在实际使用中,你可能需要添加一些额外的功能,如并发控制、过期时间等。
相关推荐
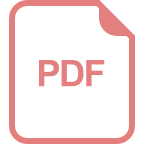
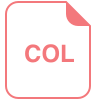













