LRU python实现
时间: 2023-12-04 21:37:40 浏览: 136
LRU(Least Recently Used)算法是一种常用的缓存淘汰策略,用于在有限的缓存空间内管理最近访问的数据。Python实现LRU算法的方法有两种,分别是用OrderedDict实现和用dict+list实现。
用OrderedDict实现LRU算法的代码如下:
```python
from collections import OrderedDict
class LRUCache:
def __init__(self, capacity: int):
self.capacity = capacity
self.cache = OrderedDict()
def get(self, key: int) -> int:
if key not in self.cache:
return -1
self.cache.move_to_end(key)
return self.cache[key]
def put(self, key: int, value: int) -> None:
if key in self.cache:
self.cache.move_to_end(key)
self.cache[key] = value
if len(self.cache) > self.capacity:
self.cache.popitem(last=False)
```
用dict+list实现LRU算法的代码如下:
```python
class LRUCache:
def __init__(self, capacity: int):
self.capacity = capacity
self.cache = {}
self.key_list = []
def get(self, key: int) -> int:
if key not in self.cache:
return -1
self.key_list.remove(key)
self.key_list.append(key)
return self.cache[key]
def put(self, key: int, value: int) -> None:
if key in self.cache:
self.key_list.remove(key)
elif len(self.cache) == self.capacity:
del self.cache[self.key_list.pop(0)]
self.key_list.append(key)
self.cache[key] = value
```
以上两种方法都可以有效地管理缓存空间,并且具有较好的性能。你可以根据自己的需求调整缓存的容量大小,以满足实际应用的要求。
阅读全文
相关推荐
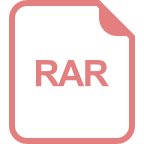

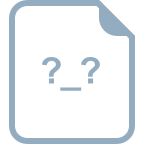
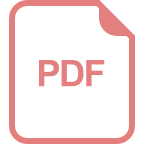
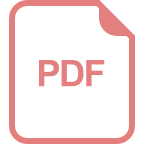
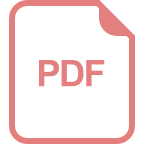
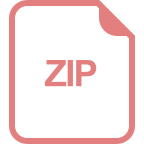
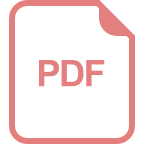
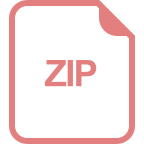
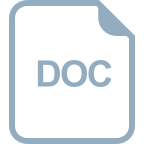
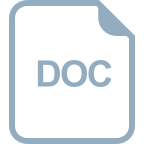
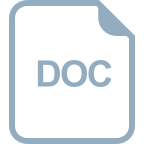
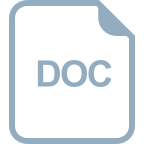
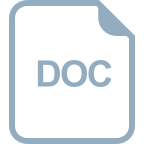
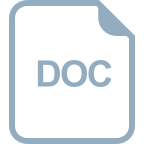
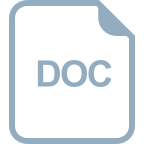
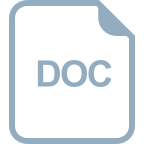