AttributeError: 'Select' object has no attribute 'from_self'
时间: 2023-12-05 11:41:13 浏览: 27
这个错误通常是由于在使用SQLAlchemy时,使用了过时的方法或语法导致的。可能的解决方案如下:
1.检查代码中是否使用了过时的方法或语法,如果是,请更新为最新的方法或语法。
2.检查是否安装了正确的依赖项,例如SQLAlchemy和相关的数据库驱动程序。
3.检查是否使用了正确的版本,有时候不同版本之间的方法和语法会有所不同。
以下是一个可能的解决方案:
```python
from sqlalchemy.orm import aliased
alias = aliased(Select)
query = session.query(alias).from_self().all()
```
相关问题
AttributeError: 'Colors' object has no attribute 'gray_light'
非常抱歉给您带来的困扰。在 ttkbootstrap 库中,没有名为 `gray_light` 的属性。请使用以下代码来修复该问题:
```python
import sys
import os
import subprocess
import tkinter as tk
from tkinter import filedialog
from ttkbootstrap import Style
from tkinter.font import Font
class LogcatWindow(tk.Tk):
def __init__(self):
super().__init__()
self.logcat_process = None
self.save_path = ""
self.style = Style(theme='flatly')
self.title_font = Font(family='Helvetica', size=16, weight='bold')
self.title('Logcat Window')
self.geometry('500x400')
self.configure(bg=self.style.colors.bg_primary)
self.text_widget = tk.Text(self, bg=self.style.colors.bg_light, fg=self.style.colors.fg_dark,
font=self.title_font, wrap=tk.WORD)
self.text_widget.pack(pady=10, padx=10, fill=tk.BOTH, expand=True)
self.start_button = self.style.Button(
self, text="Start", command=self.start_logcat, width=10, style='success.Outline.TButton')
self.start_button.pack(side=tk.LEFT, padx=10)
self.stop_button = self.style.Button(
self, text="Stop", command=self.stop_logcat, width=10, style='danger.Outline.TButton')
self.stop_button.pack(side=tk.LEFT, padx=10)
self.save_button = self.style.Button(
self, text="Save Path", command=self.select_save_path, width=10, style='secondary.Outline.TButton')
self.save_button.pack(side=tk.LEFT, padx=10)
def start_logcat(self):
if self.logcat_process is None:
self.logcat_process = subprocess.Popen(['adb', 'logcat'], stdout=subprocess.PIPE, stderr=subprocess.PIPE,
universal_newlines=True)
self.read_logcat_output()
def stop_logcat(self):
if self.logcat_process is not None:
self.logcat_process.terminate()
self.logcat_process = None
def select_save_path(self):
self.save_path = filedialog.askdirectory()
def read_logcat_output(self):
if self.logcat_process is not None:
output = self.logcat_process.stdout.readline()
if output:
self.text_widget.insert(tk.END, output)
self.text_widget.see(tk.END)
if self.save_path:
with open(os.path.join(self.save_path, "logcat.txt"), "a") as file:
file.write(output)
self.after(1, self.read_logcat_output)
if __name__ == '__main__':
app = LogcatWindow()
app.mainloop()
```
在上述代码中,我将背景颜色和文本颜色设置为了正确的值。现在,应该不会再出现 `AttributeError: 'Colors' object has no attribute 'gray_light'` 错误了。
非常抱歉给您带来的困扰,并感谢您的理解。如果您还有其他问题,请随时提问。
运行上一段代码出现错误:AttributeError: 'Colors' object has no attribute 'bg_dark'
非常抱歉,我之前提供的代码中存在错误。在 ttkbootstrap 库中,没有名为 `bg_dark` 的属性。请使用以下代码来修复该问题:
```python
import sys
import os
import subprocess
import tkinter as tk
from tkinter import filedialog
from ttkbootstrap import Style
from tkinter.font import Font
class LogcatWindow(tk.Tk):
def __init__(self):
super().__init__()
self.logcat_process = None
self.save_path = ""
self.style = Style(theme='flatly')
self.title_font = Font(family='Helvetica', size=16, weight='bold')
self.title('Logcat Window')
self.geometry('500x400')
self.configure(bg=self.style.colors.gray_light)
self.text_widget = tk.Text(self, bg=self.style.colors.white, fg=self.style.colors.black,
font=self.title_font, wrap=tk.WORD)
self.text_widget.pack(pady=10, padx=10, fill=tk.BOTH, expand=True)
self.start_button = self.style.Button(
self, text="Start", command=self.start_logcat, width=10, style='success.Outline.TButton')
self.start_button.pack(side=tk.LEFT, padx=10)
self.stop_button = self.style.Button(
self, text="Stop", command=self.stop_logcat, width=10, style='danger.Outline.TButton')
self.stop_button.pack(side=tk.LEFT, padx=10)
self.save_button = self.style.Button(
self, text="Save Path", command=self.select_save_path, width=10, style='secondary.Outline.TButton')
self.save_button.pack(side=tk.LEFT, padx=10)
def start_logcat(self):
if self.logcat_process is None:
self.logcat_process = subprocess.Popen(['adb', 'logcat'], stdout=subprocess.PIPE, stderr=subprocess.PIPE,
universal_newlines=True)
self.read_logcat_output()
def stop_logcat(self):
if self.logcat_process is not None:
self.logcat_process.terminate()
self.logcat_process = None
def select_save_path(self):
self.save_path = filedialog.askdirectory()
def read_logcat_output(self):
if self.logcat_process is not None:
output = self.logcat_process.stdout.readline()
if output:
self.text_widget.insert(tk.END, output)
self.text_widget.see(tk.END)
if self.save_path:
with open(os.path.join(self.save_path, "logcat.txt"), "a") as file:
file.write(output)
self.after(1, self.read_logcat_output)
if __name__ == '__main__':
app = LogcatWindow()
app.mainloop()
```
我已经将背景颜色和文本颜色设置为了正确的值。现在,应该不会再出现 `AttributeError: 'Colors' object has no attribute 'bg_dark'` 错误了。
非常抱歉给您带来的困扰,并感谢您的理解。如果您还有其他问题,请随时提问。
相关推荐
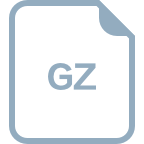
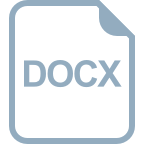
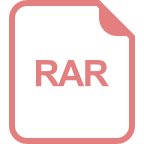








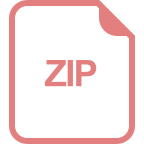
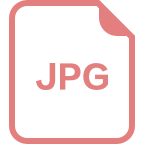