winform binding
时间: 2023-08-24 17:04:36 浏览: 164
WinForms binding 是指在 Windows Forms 应用程序中使用数据绑定的技术。它可以将数据源与 Windows 控件进行关联,实现数据的自动更新和同步。
要在 WinForms 中进行数据绑定,你需要以下几个步骤:
1. 创建一个数据源:可以是一个集合(如 List、DataTable),或一个对象(如实体类)。
2. 在窗体上添加控件,如 TextBox、ComboBox、DataGridView 等。
3. 设置控件的 DataBindings 属性,指定要绑定到的数据源和字段。
4. 可选:设置控件的格式化、验证和更新模式等属性,以满足特定需求。
5. 运行应用程序,控件将自动显示和更新数据源中的数据。
下面是一个简单的示例代码,演示如何在 WinForms 中进行数据绑定:
```csharp
using System;
using System.Windows.Forms;
namespace WinFormsBindingExample
{
public partial class Form1 : Form
{
private BindingSource bindingSource;
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
// 创建数据源(这里使用一个简单的字符串列表)
var dataSource = new string[] { "Apple", "Banana", "Orange" };
// 创建 BindingSource 对象,并将数据源设置为该对象的 DataSource 属性
bindingSource = new BindingSource();
bindingSource.DataSource = dataSource;
// 将 BindingSource 对象与控件的 DataBindings 属性关联
listBox1.DataSource = bindingSource;
}
private void buttonAdd_Click(object sender, EventArgs e)
{
// 添加新的数据项到数据源
bindingSource.Add(textBox1.Text);
}
private void buttonRemove_Click(object sender, EventArgs e)
{
// 从数据源中移除选定的数据项
if (listBox1.SelectedIndex != -1)
{
bindingSource.RemoveAt(listBox1.SelectedIndex);
}
}
}
}
```
在上述示例中,我们创建了一个 ListBox 控件,并通过 BindingSource 对象将其与数据源(字符串列表)进行绑定。用户可以通过 TextBox 输入框输入新的数据项,并通过添加和删除按钮来操作数据源。
这只是一个简单的示例,实际上 WinForms 数据绑定可以支持更复杂的场景,如绑定到数据库、使用自定义格式化器等。你可以根据具体需求进行进一步学习和实践。
阅读全文
相关推荐
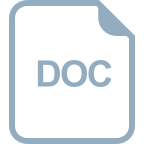
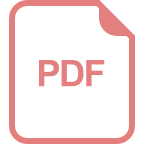
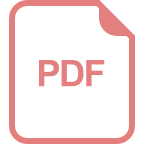
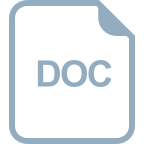

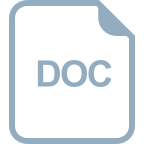
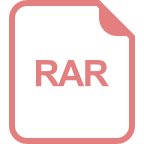
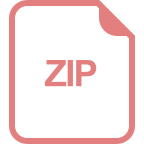
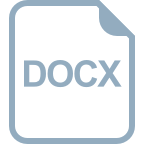
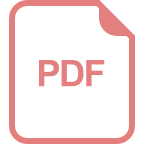
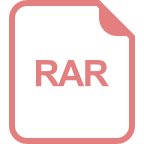
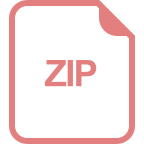
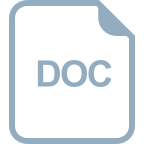


