C# StreamReader
时间: 2023-10-22 22:57:52 浏览: 146
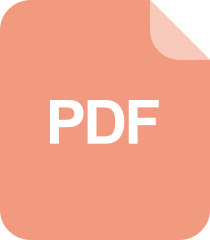
C#使用StreamReader读取文件的方法
C#中的StreamReader和StreamWriter是用于读取和写入文本文件的类。StreamReader类用于从文本文件中读取数据,而StreamWriter类用于将数据写入文本文件中。
StreamReader类提供了一些方法,如ReadLine()和ReadToEnd(),用于读取文本文件中的数据。它还提供了一些属性,如CurrentEncoding和BaseStream,用于获取当前编码和基础流。
StreamWriter类提供了一些方法,如Write()和WriteLine(),用于将数据写入文本文件中。它还提供了一些属性,如AutoFlush和BaseStream,用于自动刷新缓冲区和获取基础流。
这两个类都实现了IDisposable接口,因此应该使用using语句来确保在使用完后释放资源。
阅读全文
相关推荐
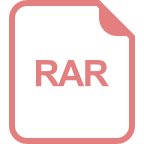














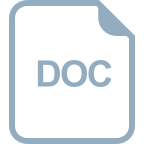