使用jq完成轮播图效果 轮播图大小为200px*200px,实现四张图片轮播 轮播图上有两个按钮,实现选择图片上一张下一张的效果 当鼠标放到图片上时,轮播停止,鼠标离开,轮播继续,请写出代码
时间: 2023-08-31 09:43:30 浏览: 79
### 回答1:
以下是使用jQuery完成轮播图效果的代码,实现了在一个200px*200px大小的轮播图中展示四张图片,并在轮播图上添加了两个按钮,实现了选取图片上一张或下一张的功能。当鼠标移到轮播图上时,轮播图暂停,当鼠标移出轮播图时,轮播图继续轮播。
HTML 代码:
```
<div id="slideshow">
<img src="image1.jpg">
<img src="image2.jpg">
<img src="image3.jpg">
<img src="image4.jpg">
<button id="prevButton">上一张</button>
<button id="nextButton">下一张</button>
</div>
```
CSS 代码:
```
#slideshow {
width: 200px;
height: 200px;
position: relative;
overflow: hidden;
}
#slideshow img {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
opacity: 0;
transition: opacity 1s ease-in-out;
}
#slideshow img.active {
opacity: 1;
}
#prevButton, #nextButton {
position: absolute;
top: 50%;
transform: translateY(-50%);
width: 50px;
height: 50px;
font-size: 20px;
color: #fff;
background-color: rgba(0, 0, 0, 0.5);
border: none;
cursor: pointer;
}
#prevButton {
left: 0;
}
#nextButton {
right: 0;
}
```
JavaScript 代码:
```
$(document).ready(function() {
var slideIndex = 1;
showSlides(slideIndex);
var slideTimer = setInterval(function() {
slideIndex++;
showSlides(slideIndex);
}, 2000);
$("#prevButton").click(function() {
clearInterval(slideTimer);
slideIndex--;
showSlides(slideIndex);
});
$("#nextButton").click(function() {
clearInterval(slideTimer);
slideIndex++;
showSlides(slideIndex);
});
$("#slideshow").mouseenter(function() {
clearInterval(slideTimer);
});
$("#slideshow").mouseleave(function() {
slideTimer = setInterval(function() {
slideIndex++;
showSlides(slideIndex);
}, 2000);
});
function showSlides(n) {
var slides = $("#slideshow img");
if (n > slides.length) {
slideIndex = 1;
}
if (n < 1) {
slideIndex = slides.length;
}
slides.removeClass("active");
slides.eq(slideIndex - 1).addClass("active");
}
});
```
### 回答2:
使用jq完成轮播图效果,代码如下:
HTML部分:
```html
<div class="carousel">
<div class="img-container">
<img src="image1.jpg" alt="Image 1" width="200px" height="200px">
<img src="image2.jpg" alt="Image 2" width="200px" height="200px">
<img src="image3.jpg" alt="Image 3" width="200px" height="200px">
<img src="image4.jpg" alt="Image 4" width="200px" height="200px">
</div>
<div class="controls">
<button class="prev-btn">上一张</button>
<button class="next-btn">下一张</button>
</div>
</div>
```
CSS部分:
```css
.carousel {
position: relative;
width: 200px;
height: 200px;
overflow: hidden;
}
.img-container {
width: 800px; /* 轮播图总宽度为每张图片宽度的4倍 */
height: 200px;
display: flex;
}
.img-container img {
width: 200px;
height: 200px;
}
.controls {
position: absolute;
bottom: 0;
width: 200px;
text-align: center;
}
.prev-btn, .next-btn {
margin: 10px;
}
```
JavaScript部分:
```javascript
$(document).ready(function() {
var carouselInterval;
function startCarousel() {
carouselInterval = setInterval(function() {
var $currentImg = $('.img-container img:first');
var $nextImg = $currentImg.next();
$currentImg.animate({left: '-200px'}, 1000, function(){
$currentImg.appendTo('.img-container').css('left', '');
});
$nextImg.css('left', '200px');
}, 3000);
}
$('.prev-btn').click(function() {
var $currentImg = $('.img-container img:first');
var $prevImg = $('.img-container img:last');
$prevImg.prependTo('.img-container').css('left', '-200px');
$currentImg.animate({left: '200px'}, 1000, function(){
$currentImg.css('left', '');
});
});
$('.next-btn').click(function() {
var $currentImg = $('.img-container img:first');
var $nextImg = $currentImg.next();
$currentImg.animate({left: '-200px'}, 1000, function(){
$currentImg.appendTo('.img-container').css('left', '');
});
$nextImg.css('left', '200px');
});
$('.carousel').mouseenter(function() {
clearInterval(carouselInterval);
}).mouseleave(function() {
startCarousel();
});
startCarousel();
});
```
以上代码实现了一个轮播图效果,轮播图大小为200px*200px,共有四张图片进行轮播。页面上有两个按钮,分别用于选择上一张和下一张图片。当鼠标放置在轮播图上时,轮播暂停,当鼠标离开时,轮播继续。
### 回答3:
使用jq完成轮播图效果可以通过以下代码实现:
HTML部分:
```html
<div class="carousel-container">
<div class="carousel">
<img src="image1.jpg" alt="Image 1">
<img src="image2.jpg" alt="Image 2">
<img src="image3.jpg" alt="Image 3">
<img src="image4.jpg" alt="Image 4">
</div>
<div class="controls">
<button class="prev-btn">上一张</button>
<button class="next-btn">下一张</button>
</div>
</div>
```
CSS部分:
```css
.carousel-container {
position: relative;
width: 200px;
height: 200px;
overflow: hidden;
}
.carousel {
position: absolute;
width: 800px; /* 200px * 4 */
height: 200px;
}
.carousel img {
width: 200px;
height: 200px;
}
.controls {
position: absolute;
bottom: 0;
width: 100%;
text-align: center;
}
.prev-btn, .next-btn {
padding: 5px 10px;
margin: 5px;
}
```
JavaScript部分:
```javascript
$(document).ready(function() {
var carousel = $('.carousel');
var images = carousel.find('img');
var imageWidth = carousel.width() / images.length;
var currentIndex = 0;
var timer = null;
// 设置轮播图和按钮的宽度
images.width(imageWidth);
$('.controls button').width(imageWidth);
// 轮播函数
function startCarousel() {
timer = setInterval(function() {
currentIndex = (currentIndex + 1) % images.length;
carousel.animate({
marginLeft: -imageWidth * currentIndex
});
}, 2000); // 每隔2秒切换一张图
}
// 开始轮播
startCarousel();
// 图片上一张按钮点击事件
$('.prev-btn').click(function() {
clearInterval(timer);
currentIndex = (currentIndex - 1 + images.length) % images.length;
carousel.animate({
marginLeft: -imageWidth * currentIndex
});
startCarousel();
});
// 图片下一张按钮点击事件
$('.next-btn').click(function() {
clearInterval(timer);
currentIndex = (currentIndex + 1) % images.length;
carousel.animate({
marginLeft: -imageWidth * currentIndex
});
startCarousel();
});
// 鼠标移入停止轮播,移出继续轮播
carousel.hover(function() {
clearInterval(timer);
}, function() {
startCarousel();
});
});
```
以上代码实现了一个简单的轮播图效果,轮播图的大小为200px * 200px,共有四张图片进行轮播。轮播图上方包含上一张和下一张两个按钮,点击按钮可切换图片。当鼠标放在图片上时,轮播图停止,鼠标离开时轮播图继续。
相关推荐
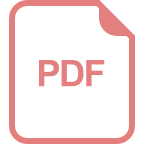
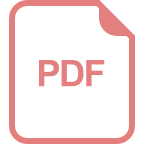
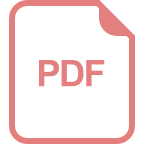














