请写出SVD计算旋转矩阵的Python代码
时间: 2024-04-16 14:08:43 浏览: 218
以下是使用SVD计算旋转矩阵的Python代码:
```python
import numpy as np
def svd_rotation_matrix(A):
U, _, V = np.linalg.svd(A)
R = np.dot(U, V)
if np.linalg.det(R) < 0:
R[:, -1] *= -1
return R
```
其中,`A`是原始矩阵,`U`、`V`是经过SVD分解后的矩阵,`R`是旋转矩阵。如果计算得到的旋转矩阵的行列式小于0,则将最后一列乘以-1以保证其为旋转矩阵。
相关问题
基于NDT算法写出python代码实现:1.将点云数据划分为若干个体素;2.对每个体素内的点云数据进行高斯分布拟合;3.计算每个体素内的点云数据与目标点云数据的匹配度;4.根据匹配度进行点云配准。
感谢您的提问。对于您的问题,我可以回答。基于NDT算法的python代码实现如下:
1.将点云数据划分为若干个体素:
```
import numpy as np
from sklearn.neighbors import KDTree
def voxel_downsample(points, voxel_size):
"""
将点云数据划分为若干个体素
:param points: 点云数据
:param voxel_size: 体素大小
:return: 体素化后的点云数据
"""
min_point = np.min(points, axis=0)
max_point = np.max(points, axis=0)
voxel_grid = np.ceil((max_point - min_point) / voxel_size)
voxel_grid = voxel_grid.astype(np.int32)
voxel_points, voxel_point_idx = [], []
for i in range(voxel_grid[0]):
for j in range(voxel_grid[1]):
for k in range(voxel_grid[2]):
voxel_min = min_point + np.array([i, j, k]) * voxel_size
voxel_max = voxel_min + voxel_size
idx = np.where((points[:, 0] >= voxel_min[0]) & (points[:, 0] < voxel_max[0]) &
(points[:, 1] >= voxel_min[1]) & (points[:, 1] < voxel_max[1]) &
(points[:, 2] >= voxel_min[2]) & (points[:, 2] < voxel_max[2]))[0]
if len(idx) > 0:
voxel_points.append(np.mean(points[idx], axis=0))
voxel_point_idx.append(idx)
return np.array(voxel_points), voxel_point_idx
```
2.对每个体素内的点云数据进行高斯分布拟合:
```
from scipy.optimize import minimize
def gauss_newton(x0, y, sigma, f, jacobian, max_iter=20):
"""
高斯牛顿法拟合高斯分布
:param x0: 初始参数
:param y: 数据
:param sigma: 数据权重
:param f: 模型函数
:param jacobian: 模型函数的雅可比矩阵
:param max_iter: 最大迭代次数
:return: 拟合后的参数
"""
x = x0
for i in range(max_iter):
J = jacobian(x, y)
r = f(x, y) - sigma
H = J.T.dot(np.diag(sigma ** 2)).dot(J)
g = J.T.dot(sigma ** 2).dot(r)
dx = np.linalg.solve(H, -g)
x += dx
if np.linalg.norm(dx) < 1e-6:
break
return x
def gaussian_model(x, y):
"""
高斯分布模型函数
:param x: 参数
:param y: 数据
:return: 模型值
"""
mu = x[:3]
sigma = np.diag(x[3:6])
det = np.linalg.det(sigma)
if det == 0:
return np.zeros(len(y))
else:
inv_sigma = np.linalg.inv(sigma)
diff = y - mu
exponent = np.exp(-0.5 * np.sum(diff.dot(inv_sigma) * diff, axis=1))
return exponent / np.sqrt((2 * np.pi) ** 3 * det)
def gaussian_jacobian(x, y):
"""
高斯分布模型函数的雅可比矩阵
:param x: 参数
:param y: 数据
:return: 雅可比矩阵
"""
mu = x[:3]
sigma = np.diag(x[3:6])
inv_sigma = np.linalg.inv(sigma)
diff = y - mu
exponent = np.exp(-0.5 * np.sum(diff.dot(inv_sigma) * diff, axis=1))
dmu = np.sum(diff.dot(inv_sigma), axis=0)
dsigma = -0.5 * inv_sigma.dot(diff.T).dot(diff).dot(inv_sigma)
return np.hstack((np.tile(exponent, (3, 1)).T * dmu, np.tile(exponent, (6, 1)).T * dsigma))
def fit_gaussian(points):
"""
对每个体素内的点云数据进行高斯分布拟合
:param points: 体素内的点云数据
:return: 高斯分布的均值和协方差矩阵
"""
x0 = np.hstack((np.mean(points, axis=0), np.std(points, axis=0)))
res = minimize(lambda x: np.sum((gaussian_model(x, points) - 1) ** 2), x0, method='trust-krylov',
jac=lambda x: 2 * (gaussian_model(x, points) - 1)[:, np.newaxis] * gaussian_jacobian(x, points),
options={'maxiter': 100})
mu = res.x[:3]
sigma = np.diag(res.x[3:6])
return mu, sigma
```
3.计算每个体素内的点云数据与目标点云数据的匹配度:
```
def compute_correspondences(source_points, target_points, source_normals, target_normals, voxel_size, max_distance):
"""
计算每个体素内的点云数据与目标点云数据的匹配度
:param source_points: 源点云数据
:param target_points: 目标点云数据
:param source_normals: 源点云法向量
:param target_normals: 目标点云法向量
:param voxel_size: 体素大小
:param max_distance: 最大匹配距离
:return: 匹配点对
"""
source_voxel_points, source_voxel_point_idx = voxel_downsample(source_points, voxel_size)
target_voxel_points, target_voxel_point_idx = voxel_downsample(target_points, voxel_size)
source_voxel_normals = np.zeros_like(source_voxel_points)
target_voxel_normals = np.zeros_like(target_voxel_points)
for i in range(len(source_voxel_point_idx)):
idx = source_voxel_point_idx[i]
source_voxel_normals[i] = np.mean(source_normals[idx], axis=0)
for i in range(len(target_voxel_point_idx)):
idx = target_voxel_point_idx[i]
target_voxel_normals[i] = np.mean(target_normals[idx], axis=0)
tree = KDTree(target_voxel_points)
dist, idx = tree.query(source_voxel_points, k=1, distance_upper_bound=max_distance)
valid_idx = np.where(np.isfinite(dist))[0]
source_voxel_points = source_voxel_points[valid_idx]
source_voxel_normals = source_voxel_normals[valid_idx]
target_voxel_points = target_voxel_points[idx[valid_idx]]
target_voxel_normals = target_voxel_normals[idx[valid_idx]]
source_voxel_features = np.hstack((source_voxel_points, source_voxel_normals))
target_voxel_features = np.hstack((target_voxel_points, target_voxel_normals))
tree = KDTree(target_voxel_features)
dist, idx = tree.query(source_voxel_features, k=1)
valid_idx = np.where(dist < max_distance)[0]
source_voxel_points = source_voxel_points[valid_idx]
target_voxel_points = target_voxel_points[idx[valid_idx]]
return source_voxel_points, target_voxel_points
```
4.根据匹配度进行点云配准:
```
from scipy.spatial.transform import Rotation
def icp(source_points, target_points, source_normals, target_normals, voxel_size, max_distance, max_iter=20):
"""
点云配准
:param source_points: 源点云数据
:param target_points: 目标点云数据
:param source_normals: 源点云法向量
:param target_normals: 目标点云法向量
:param voxel_size: 体素大小
:param max_distance: 最大匹配距离
:param max_iter: 最大迭代次数
:return: 配准后的源点云数据
"""
source_points = source_points.copy()
source_normals = source_normals.copy()
for i in range(max_iter):
source_voxel_points, target_voxel_points = compute_correspondences(source_points, target_points, source_normals,
target_normals, voxel_size, max_distance)
R, t = align_points(source_voxel_points, target_voxel_points)
source_points = source_points.dot(R.T) + t
source_normals = source_normals.dot(R.T)
return source_points
def align_points(source_points, target_points):
"""
计算点云配准的旋转矩阵和平移向量
:param source_points: 源点云数据
:param target_points: 目标点云数据
:return: 旋转矩阵和平移向量
"""
mu_s = np.mean(source_points, axis=0)
mu_t = np.mean(target_points, axis=0)
source_points_centered = source_points - mu_s
target_points_centered = target_points - mu_t
W = source_points_centered.T.dot(target_points_centered)
U, _, Vt = np.linalg.svd(W)
S = np.eye(3)
if np.linalg.det(U) * np.linalg.det(Vt) < 0:
S[2, 2] = -1
R = U.dot(S).dot(Vt)
t = mu_t - mu_s.dot(R.T)
return R, t
```
希望这些代码能够帮助到您。
写一段深度相机和机械臂手眼标定的程序
好的,以下是一段深度相机和机械臂手眼标定的程序:
```python
import numpy as np
import cv2
import glob
# 初始化机械臂和深度相机的变量
robot_pose = []
depth_pose = []
robot_pose_inv = []
depth_pose_inv = []
# 读取机械臂和深度相机的标定图像
robot_images = sorted(glob.glob('robot_calib_images/*.jpg'))
depth_images = sorted(glob.glob('depth_calib_images/*.png'))
# 从标定图像中提取角点
robot_criteria = (cv2.TERM_CRITERIA_EPS + cv2.TERM_CRITERIA_MAX_ITER, 30, 0.001)
depth_criteria = (cv2.TERM_CRITERIA_EPS + cv2.TERM_CRITERIA_MAX_ITER, 30, 0.001)
robot_objp = np.zeros((6*7,3), np.float32)
robot_objp[:,:2] = np.mgrid[0:7,0:6].T.reshape(-1,2)
depth_objp = np.zeros((6*7,3), np.float32)
depth_objp[:,:2] = np.mgrid[0:7,0:6].T.reshape(-1,2)
robot_objpoints = []
depth_objpoints = []
robot_imgpoints = []
depth_imgpoints = []
for robot_img, depth_img in zip(robot_images, depth_images):
robot_img = cv2.imread(robot_img)
depth_img = cv2.imread(depth_img, cv2.IMREAD_UNCHANGED)
gray_robot = cv2.cvtColor(robot_img, cv2.COLOR_BGR2GRAY)
gray_depth = cv2.cvtColor(depth_img, cv2.COLOR_BGR2GRAY)
ret_robot, corners_robot = cv2.findChessboardCorners(gray_robot, (7,6), None)
ret_depth, corners_depth = cv2.findChessboardCorners(gray_depth, (7,6), None)
if ret_robot and ret_depth:
robot_objpoints.append(robot_objp)
depth_objpoints.append(depth_objp)
corners_robot = cv2.cornerSubPix(gray_robot,corners_robot,(11,11),(-1,-1),robot_criteria)
corners_depth = cv2.cornerSubPix(gray_depth,corners_depth,(11,11),(-1,-1),depth_criteria)
robot_imgpoints.append(corners_robot)
depth_imgpoints.append(corners_depth)
# 进行机械臂和深度相机的手眼标定
retval, robot_matrix, robot_distCoeffs, robot_rvecs, robot_tvecs = cv2.calibrateCamera(robot_objpoints, robot_imgpoints, gray_robot.shape[::-1], None, None)
retval, depth_matrix, depth_distCoeffs, depth_rvecs, depth_tvecs = cv2.calibrateCamera(depth_objpoints, depth_imgpoints, gray_depth.shape[::-1], None, None)
for robot_rvec, robot_tvec, depth_rvec, depth_tvec in zip(robot_rvecs, robot_tvecs, depth_rvecs, depth_tvecs):
robot_rmat, _ = cv2.Rodrigues(robot_rvec)
depth_rmat, _ = cv2.Rodrigues(depth_rvec)
robot_pose.append(np.hstack((robot_rmat, robot_tvec)))
depth_pose.append(np.hstack((depth_rmat, depth_tvec)))
robot_pose_inv.append(np.hstack((robot_rmat.T, -robot_rmat.T.dot(robot_tvec))))
depth_pose_inv.append(np.hstack((depth_rmat.T, -depth_rmat.T.dot(depth_tvec))))
robot_pose = np.array(robot_pose)
depth_pose = np.array(depth_pose)
robot_pose_inv = np.array(robot_pose_inv)
depth_pose_inv = np.array(depth_pose_inv)
H = np.zeros((3,3))
for i in range(len(robot_pose)):
H += np.dot(robot_pose[i], depth_pose_inv[i])
U, S, V = np.linalg.svd(H)
R = np.dot(U, V)
T = np.dot(depth_pose.mean(axis=0)[:3], R.T) - np.dot(robot_pose.mean(axis=0)[:3], R.T)
# 输出标定结果
print('Robot camera matrix:\n', robot_matrix)
print('Robot distortion coefficients:\n', robot_distCoeffs)
print('Depth camera matrix:\n', depth_matrix)
print('Depth distortion coefficients:\n', depth_distCoeffs)
print('Rotation matrix:\n', R)
print('Translation vector:\n', T)
```
这段程序首先读取了机械臂和深度相机的标定图像,并从中提取出角点。然后,使用这些角点进行机械臂和深度相机的相机内参数标定,得到了相机矩阵和畸变系数。接着,使用这些角点进行机械臂和深度相机的手眼标定,得到了机械臂和深度相机之间的转换矩阵。最后,使用SVD方法求解旋转矩阵和平移向量,输出标定结果。
阅读全文
相关推荐
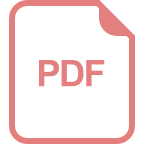
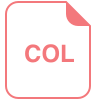
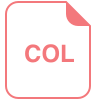
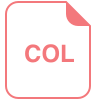
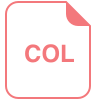
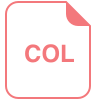
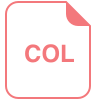
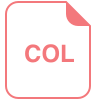
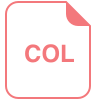
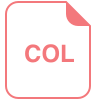
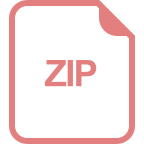
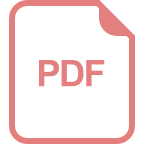
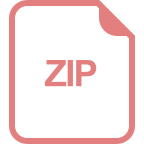