用Python写一个凯撒密码
时间: 2023-04-01 19:01:17 浏览: 108
凯撒密码是一种简单的加密方法,可以通过将明文中的每个字母按照一定的偏移量进行替换来得到密文。以下是用 Python 实现凯撒密码的代码:
```python
def caesar_cipher(text, shift):
result = ""
for char in text:
if char.isalpha():
if char.isupper():
result += chr((ord(char) + shift - 65) % 26 + 65)
else:
result += chr((ord(char) + shift - 97) % 26 + 97)
else:
result += char
return result
```
其中,`text` 是要加密的明文,`shift` 是偏移量。这个函数会返回加密后的密文。
例如,如果要将明文 `"hello, world!"` 用偏移量为 3 的凯撒密码进行加密,可以这样调用函数:
```python
cipher_text = caesar_cipher("hello, world!", 3)
print(cipher_text) # "khoor, zruog!"
```
这样就得到了密文 `"khoor, zruog!"`。
相关问题
用python编写一个凯撒密码加密算法
好的,以下是用 Python 编写的凯撒密码加密算法示例:
```python
def caesar_encrypt(plain_text, shift):
"""凯撒密码加密函数"""
cipher_text = ""
for char in plain_text:
if char.isalpha():
# 对字母进行加密
ascii_code = ord(char) + shift
if char.isupper():
if ascii_code > ord('Z'):
ascii_code -= 26
elif ascii_code < ord('A'):
ascii_code += 26
else:
if ascii_code > ord('z'):
ascii_code -= 26
elif ascii_code < ord('a'):
ascii_code += 26
cipher_text += chr(ascii_code)
else:
# 非字母字符直接添加到密文中
cipher_text += char
return cipher_text
```
这个函数接收两个参数,第一个参数 plain_text 是要加密的明文,第二个参数 shift 是加密时的位移量。函数会遍历明文中的每个字符,对字母进行加密,非字母字符则直接添加到密文中。字母加密时会根据大小写分别处理,并根据位移量进行加密。
下面是一个示例,使用该函数进行加密:
```python
plain_text = "hello, world!"
shift = 3
cipher_text = caesar_encrypt(plain_text, shift)
print(cipher_text)
```
输出结果为:
```
khoor, zruog!
```
用Python写凯撒密码
凯撒密码是一种简单的加密算法,它将明文中的每个字母都按照一定的偏移量进行移位,从而得到密文。在Python中,我们可以用以下代码实现凯撒密码:
```python
def caesar_cipher(text, shift):
"""
凯撒密码加密算法实现
"""
result = ""
for char in text:
if char.isalpha():
# 对字母进行移位操作
ascii_code = ord(char) + shift
if char.isupper():
if ascii_code > ord('Z'):
ascii_code -= 26
elif ascii_code < ord('A'):
ascii_code += 26
elif char.islower():
if ascii_code > ord('z'):
ascii_code -= 26
elif ascii_code < ord('a'):
ascii_code += 26
result += chr(ascii_code)
else:
# 非字母字符直接拼接
result += char
return result
```
其中,`text`表示要加密的明文字符串,`shift`表示偏移量,也就是每个字母要移动的距离。函数的返回值为加密后的密文字符串。
例如,我们可以这样调用函数进行加密:
```python
text = "Hello, World!"
shift = 3
cipher_text = caesar_cipher(text, shift)
print(cipher_text)
```
输出结果为:
```
Khoor, Zruog!
```
这就是通过凯撒密码算法对明文进行加密后得到的密文。
相关推荐
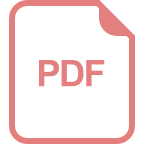
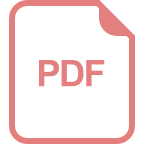
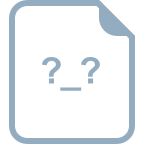
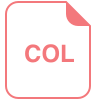
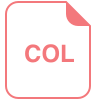
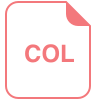









