请写出传入一帧yuyv数据,转换成I420格式后并存放到x264_picture_t的img.panel中,用C语言写出程序
时间: 2024-03-04 18:52:20 浏览: 76
以下是一个简单的C语言程序,实现了将YUYV格式数据转换成I420格式,并将其存放到x264_picture_t结构体变量的img.panel成员中。
```c
#include <stdlib.h>
#include <stdint.h>
#include <x264.h>
void yuyv_to_i420(uint8_t *src, uint8_t *dst, int width, int height)
{
int i, j;
uint8_t *y, *u, *v;
uint8_t *y_plane, *u_plane, *v_plane;
y = dst;
u = dst + width * height;
v = u + (width * height / 4);
y_plane = src;
u_plane = src + 1;
v_plane = src + 3;
for(i = 0; i < height; i++)
{
for(j = 0; j < width; j += 2)
{
*y++ = *y_plane++;
*y++ = *(y_plane + 2);
*u++ = (*u_plane + *(u_plane + 2)) / 2;
*v++ = (*v_plane + *(v_plane + 2)) / 2;
y_plane += 4;
u_plane += 4;
v_plane += 4;
}
}
}
int main()
{
uint8_t *src_data; // YUYV格式数据
uint8_t *dst_data; // I420格式数据
int width, height; // 图像宽度和高度
// 假设已经从某个地方读取到了YUYV格式的图像数据
// 并将其存储到了src_data中,同时也已经获取到了图像的宽度和高度
// 分配内存保存转换后的I420格式图像数据
dst_data = (uint8_t*)malloc(width * height * 3 / 2);
// 将YUYV格式数据转换成I420格式
yuyv_to_i420(src_data, dst_data, width, height);
// 将转换后的数据存储到x264_picture_t结构体变量的img.panel成员中
x264_picture_t picture;
picture.img.i_stride[0] = width;
picture.img.i_stride[1] = width / 2;
picture.img.i_stride[2] = width / 2;
picture.img.plane[0] = dst_data;
picture.img.plane[1] = dst_data + width * height;
picture.img.plane[2] = dst_data + width * height * 5 / 4;
// 其他操作,例如使用x264编码器进行视频编码等
// 释放内存
free(dst_data);
return 0;
}
```
需要注意的是,上面的代码只是一个简单的示例,实际应用中还需要进行一些错误处理、内存管理等方面的处理。
阅读全文
相关推荐
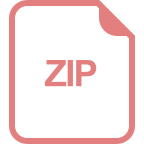
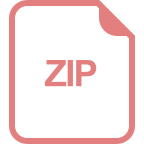
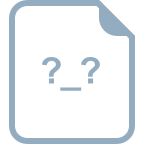















