java spark2.0实现随机森林
时间: 2023-06-30 22:06:07 浏览: 90
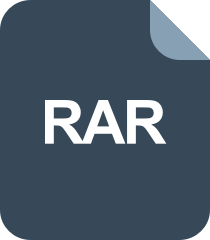
spark随机森林例子(java版)

在 Java 中,可以使用 Apache Spark 的 MLlib 库来实现随机森林。下面是一个简单的示例代码:
```java
import org.apache.spark.ml.Pipeline;
import org.apache.spark.ml.classification.RandomForestClassifier;
import org.apache.spark.ml.evaluation.MulticlassClassificationEvaluator;
import org.apache.spark.ml.feature.IndexToString;
import org.apache.spark.ml.feature.StringIndexer;
import org.apache.spark.ml.feature.VectorAssembler;
import org.apache.spark.sql.Dataset;
import org.apache.spark.sql.Row;
// 加载数据
Dataset<Row> data = spark.read().format("libsvm").load("data/sample_libsvm_data.txt");
// 将标签转换为索引
StringIndexer labelIndexer = new StringIndexer()
.setInputCol("label")
.setOutputCol("indexedLabel")
.fit(data);
// 将特征向量组合为一个特征列
VectorAssembler assembler = new VectorAssembler()
.setInputCols(new String[]{"features"})
.setOutputCol("indexedFeatures");
// 拆分数据集为训练集和测试集
Dataset<Row>[] splits = data.randomSplit(new double[]{0.7, 0.3});
Dataset<Row> trainingData = splits[0];
Dataset<Row> testData = splits[1];
// 训练随机森林模型
RandomForestClassifier rf = new RandomForestClassifier()
.setLabelCol("indexedLabel")
.setFeaturesCol("indexedFeatures")
.setNumTrees(10);
// 将索引转换为标签
IndexToString labelConverter = new IndexToString()
.setInputCol("prediction")
.setOutputCol("predictedLabel")
.setLabels(labelIndexer.labels());
// 构建 Pipeline
Pipeline pipeline = new Pipeline()
.setStages(new PipelineStage[]{labelIndexer, assembler, rf, labelConverter});
// 训练模型
PipelineModel model = pipeline.fit(trainingData);
// 测试模型并计算精度
Dataset<Row> predictions = model.transform(testData);
MulticlassClassificationEvaluator evaluator = new MulticlassClassificationEvaluator()
.setLabelCol("indexedLabel")
.setPredictionCol("prediction")
.setMetricName("accuracy");
double accuracy = evaluator.evaluate(predictions);
System.out.println("Test Error = " + (1.0 - accuracy));
```
在这个例子中,我们使用 MLlib 的随机森林分类器来训练一个模型。我们首先将标签转换为索引,然后将特征向量组合为一个特征列。我们使用随机森林分类器训练模型,然后将索引转换为标签。最后,我们使用测试数据测试模型,并计算精度。
阅读全文
相关推荐
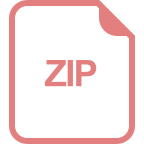

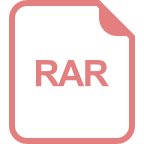
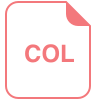
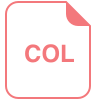
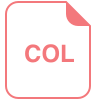
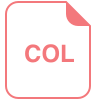
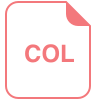
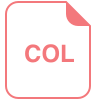
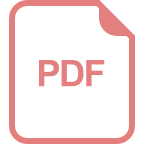
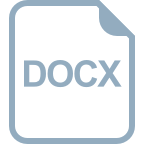