springboot 1.5 没有JasyptEncryptablePropertyPlaceholderConfigurer
时间: 2023-09-23 09:06:29 浏览: 212
### 回答1:
是的,Spring Boot 1.5中没有提供JasyptEncryptablePropertyPlaceholderConfigurer,而是使用了一个名为EncryptablePropertyPlaceholderConfigurer的类来实现属性加密。该类需要您使用Jasypt进行加密,并将密码放入Spring Boot的Environment中。以下是一个示例:
1. 添加Jasypt依赖
```xml
<dependency>
<groupId>com.github.ulisesbocchio</groupId>
<artifactId>jasypt-spring-boot</artifactId>
<version>1.17</version>
</dependency>
```
2. 在application.properties中添加加密属性
```
jasypt.encryptor.password=<your password>
```
3. 创建EncryptablePropertyPlaceholderConfigurer Bean
```java
import org.jasypt.encryption.StringEncryptor;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.PropertySource;
import org.springframework.core.env.Environment;
import org.springframework.core.io.ClassPathResource;
import org.springframework.core.io.Resource;
import org.springframework.core.io.support.EncodedResource;
import org.springframework.core.io.support.PropertiesLoaderUtils;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.util.StringUtils;
import java.io.IOException;
import java.util.Arrays;
import java.util.Properties;
@Configuration
@PropertySource(value = "classpath:application.properties")
public class PropertySourcesConfig {
@Autowired
private Environment environment;
@Autowired
private StringEncryptor encryptor;
@Bean
public static PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
@Bean
public EncryptablePropertyPlaceholderConfigurer encryptablePropertyPlaceholderConfigurer() throws IOException {
EncryptablePropertyPlaceholderConfigurer configurer = new EncryptablePropertyPlaceholderConfigurer(encryptor);
Resource[] resources = new Resource[]{
new ClassPathResource("application.properties")
};
Properties properties = null;
for (Resource resource : resources) {
PropertiesLoaderUtils.fillProperties(properties, new EncodedResource(resource, "UTF-8"));
}
configurer.setProperties(properties);
return configurer;
}
@Bean
public StringEncryptor stringEncryptor() {
return new JasyptStringEncryptor(passwordEncoder(), environment.getProperty("jasypt.encryptor.password"));
}
private static class JasyptStringEncryptor implements StringEncryptor {
private final PasswordEncoder passwordEncoder;
private final String password;
public JasyptStringEncryptor(PasswordEncoder passwordEncoder, String password) {
this.passwordEncoder = passwordEncoder;
this.password = password;
}
@Override
public String encrypt(String message) {
if (StringUtils.isEmpty(message)) {
return message;
}
return passwordEncoder.encode(message);
}
@Override
public String decrypt(String encryptedMessage) {
throw new UnsupportedOperationException("decrypt operation is not supported.");
}
}
}
```
在这个示例中,我们创建了一个名为EncryptablePropertyPlaceholderConfigurer的Bean,它使用我们之前创建的JasyptStringEncryptor来处理加密的属性。该Bean还将application.properties文件中的所有属性加载到Spring的Environment中。
### 回答2:
在SpringBoot 1.5版本中,确实没有提供JasyptEncryptablePropertyPlaceholderConfigurer。JasyptEncryptablePropertyPlaceholderConfigurer是一个用于属性加密的配置器,它使用Jasypt库将应用程序中的属性值进行加密和解密操作。
然而,SpringBoot 1.5版本提供了另一种属性加密的方法。可以通过使用@EnableEncryptableProperties注解来启用属性加密,并在application.properties文件中配置加密算法的相关信息。具体的步骤如下:
1.在项目中添加Jasypt库的依赖:
```
<dependency>
<groupId>com.github.ulisesbocchio</groupId>
<artifactId>jasypt-spring-boot-starter</artifactId>
<version>版本号</version>
</dependency>
```
2.在Spring Boot应用程序的启动类上添加@EnableEncryptableProperties注解:
```
@SpringBootApplication
@EnableEncryptableProperties
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
3.在application.properties文件中配置加密算法的相关信息:
```
jasypt.encryptor.algorithm=算法名称
jasypt.encryptor.password=加密秘钥
```
完成以上步骤后,在应用程序中可以使用@Value注解来注入经过加密的属性值。例如:
```
@Value("${encrypted.property}")
private String encryptedProperty;
```
这样,在获取属性值时,Spring Boot会自动解密加密的属性值,并注入到相应的字段中。
总结来说,虽然SpringBoot 1.5版本没有提供JasyptEncryptablePropertyPlaceholderConfigurer,但可以通过使用@EnableEncryptableProperties注解和配置加密算法的相关信息来实现属性加密的功能。
### 回答3:
在Spring Boot 1.5版本中,确实没有提供JasyptEncryptablePropertyPlaceholderConfigurer。JasyptEncryptablePropertyPlaceholderConfigurer是用于在Spring应用程序中使用Jasypt库对属性值进行加密和解密的配置器。
Jasypt是一个用于加密和解密的Java库,可用于保护应用程序中的敏感数据,如数据库密码、API密钥等。但是,在Spring Boot 1.5之前的版本中,JasyptEncryptablePropertyPlaceholderConfigurer被广泛使用,以在Spring应用程序中配置加密属性。
从Spring Boot 2.0版本开始,Spring Boot已经提供了更强大和易于使用的加密功能,取代了JasyptEncryptablePropertyPlaceholderConfigurer。现在,我们可以使用Spring的密钥存储(KeyStore)来管理应用程序中的加密密钥。通过配置application.properties或application.yml文件,我们可以指定加密密钥的位置,并使用它来加密和解密属性值。
要在Spring Boot 1.5版本中使用类似的加密功能,你可以自己实现一个类似的配置器。你可以创建一个定制的PropertyPlaceholderConfigurer,在加载属性文件时对加密的属性值进行解密。然后,在Spring应用程序的配置类中使用该配置器。
综上所述,尽管Spring Boot 1.5版本中没有提供JasyptEncryptablePropertyPlaceholderConfigurer,但你可以自己实现一个类似的配置器来实现属性值的加密和解密功能。但是,从Spring Boot 2.0版本开始,建议使用Spring Boot的内置加密功能来更方便地保护敏感数据。
阅读全文
相关推荐
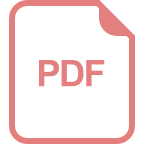
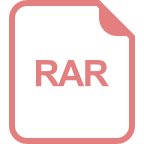
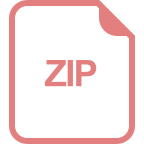
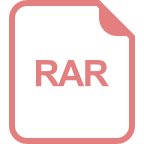
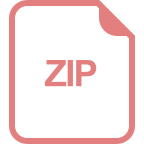
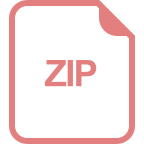
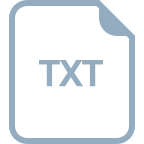
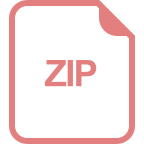
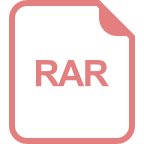
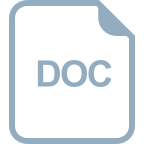
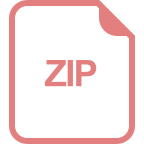
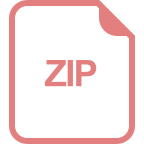
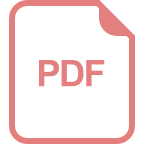