前后端分离的博客系统源码 Java
时间: 2023-08-25 22:35:00 浏览: 52
以下是一个简单的前后端分离的博客系统的 Java 代码示例,使用 Spring Boot 框架和 MySQL 数据库。
前端代码可以使用 Vue.js 或 React 等框架开发,这里不再给出示例。
### 后端代码
#### 实体类
```java
@Entity
@Table(name = "articles")
public class Article {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
private String content;
@Column(name = "created_at")
private Date createdAt;
@Column(name = "updated_at")
private Date updatedAt;
// 省略 getter 和 setter 方法
}
```
#### 数据访问层
```java
@Repository
public interface ArticleRepository extends JpaRepository<Article, Long> {
}
```
#### 服务层
```java
@Service
public class ArticleService {
@Autowired
private ArticleRepository articleRepository;
public List<Article> getAllArticles() {
return articleRepository.findAll();
}
public Article getArticleById(Long id) {
return articleRepository.findById(id)
.orElseThrow(() -> new ResourceNotFoundException("Article", "id", id));
}
public Article createArticle(Article article) {
return articleRepository.save(article);
}
public Article updateArticle(Long id, Article articleDetails) {
Article article = getArticleById(id);
article.setTitle(articleDetails.getTitle());
article.setContent(articleDetails.getContent());
article.setUpdatedAt(new Date());
return articleRepository.save(article);
}
public void deleteArticle(Long id) {
Article article = getArticleById(id);
articleRepository.delete(article);
}
}
```
#### 控制器层
```java
@RestController
@RequestMapping("/api/articles")
public class ArticleController {
@Autowired
private ArticleService articleService;
@GetMapping("")
public List<Article> getAllArticles() {
return articleService.getAllArticles();
}
@GetMapping("/{id}")
public Article getArticleById(@PathVariable(value = "id") Long id) {
return articleService.getArticleById(id);
}
@PostMapping("")
public Article createArticle(@Valid @RequestBody Article article) {
return articleService.createArticle(article);
}
@PutMapping("/{id}")
public Article updateArticle(@PathVariable(value = "id") Long id,
@Valid @RequestBody Article articleDetails) {
return articleService.updateArticle(id, articleDetails);
}
@DeleteMapping("/{id}")
public ResponseEntity<?> deleteArticle(@PathVariable(value = "id") Long id) {
articleService.deleteArticle(id);
return ResponseEntity.ok().build();
}
}
```
#### 异常处理
```java
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<?> resourceNotFoundException(ResourceNotFoundException ex) {
ErrorResponse errorResponse = new ErrorResponse(HttpStatus.NOT_FOUND.value(), ex.getMessage());
return new ResponseEntity<>(errorResponse, HttpStatus.NOT_FOUND);
}
@ExceptionHandler(MethodArgumentNotValidException.class)
public ResponseEntity<?> validationException(MethodArgumentNotValidException ex) {
ErrorResponse errorResponse = new ErrorResponse(HttpStatus.BAD_REQUEST.value(), "Validation failed");
List<String> errors = ex.getBindingResult().getFieldErrors().stream()
.map(error -> error.getField() + ": " + error.getDefaultMessage())
.collect(Collectors.toList());
errorResponse.setErrors(errors);
return new ResponseEntity<>(errorResponse, HttpStatus.BAD_REQUEST);
}
@ExceptionHandler(Exception.class)
public ResponseEntity<?> defaultExceptionHandler(Exception ex) {
ErrorResponse errorResponse = new ErrorResponse(HttpStatus.INTERNAL_SERVER_ERROR.value(), "Internal server error");
return new ResponseEntity<>(errorResponse, HttpStatus.INTERNAL_SERVER_ERROR);
}
}
```
#### 异常类
```java
public class ResourceNotFoundException extends RuntimeException {
private String resourceName;
private String fieldName;
private Object fieldValue;
public ResourceNotFoundException(String resourceName, String fieldName, Object fieldValue) {
super(String.format("%s not found with %s : '%s'", resourceName, fieldName, fieldValue));
this.resourceName = resourceName;
this.fieldName = fieldName;
this.fieldValue = fieldValue;
}
public String getResourceName() {
return resourceName;
}
public String getFieldName() {
return fieldName;
}
public Object getFieldValue() {
return fieldValue;
}
}
```
#### 错误响应类
```java
public class ErrorResponse {
private int status;
private String message;
private List<String> errors;
public ErrorResponse(int status, String message) {
this.status = status;
this.message = message;
}
public int getStatus() {
return status;
}
public void setStatus(int status) {
this.status = status;
}
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
public List<String> getErrors() {
return errors;
}
public void setErrors(List<String> errors) {
this.errors = errors;
}
}
```
### 配置文件
#### application.properties
```properties
spring.datasource.url=jdbc:mysql://localhost:3306/blog?useSSL=false&characterEncoding=utf8&serverTimezone=GMT%2B8
spring.datasource.username=root
spring.datasource.password=root
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
spring.jpa.hibernate.ddl-auto=update
spring.jpa.show-sql=true
spring.jpa.properties.hibernate.format_sql=true
```
### 总结
以上是一个简单的前后端分离的博客系统的 Java 代码示例,该示例使用了 Spring Boot 框架、MySQL 数据库和 JPA 数据访问层。该示例中,实体类、数据访问层、服务层和控制器层分别处理了数据的持久化、业务逻辑和 RESTful API 接口的实现,并使用了全局异常处理来处理异常情况。
相关推荐
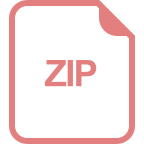
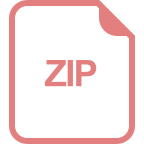
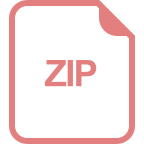














