def make_layers(c_in, c_out, repeat_times, is_downsample=False): blocks = [] for i in range(repeat_times): if i == 0: blocks += [BasicBlock(c_in, c_out, is_downsample=is_downsample), ] else: blocks += [BasicBlock(c_out, c_out), ] return nn.Sequential(*blocks)
时间: 2024-04-02 17:35:55 浏览: 131
这段代码是一个辅助函数,用于构造由多个 BasicBlock 组成的层。函数接受四个参数:
- `c_in`:输入通道数。
- `c_out`:输出通道数。
- `repeat_times`:BasicBlock 的重复次数。
- `is_downsample`:是否进行下采样(即是否需要改变输入输出的尺寸)。
在函数内部,通过循环来构造 BasicBlock 的序列,将这些 BasicBlock 依次添加到一个空列表 `blocks` 中。在第一次循环时,调用 BasicBlock 的构造函数时需要传入 `is_downsample` 参数;在后续的循环中,由于不再需要下采样,就不需要指定 `is_downsample` 参数了。最后,通过 `nn.Sequential` 将 `blocks` 列表中的 BasicBlock 组成一个序列,并返回这个序列。
相关问题
def create_decoder_block(in_channels, out_channels, kernel_size, wn=True, bn=True, activation=nn.ReLU, layers=2, final_layer=False): decoder = [] for i in range(layers): _in = in_channels _out = in_channels _bn = bn _activation = activation if i == 0: _in = in_channels * 2 if i == layers - 1: _out = out_channels if final_layer: _bn = False _activation = None decoder.append(create_layer(_in, _out, kernel_size, wn, _bn, _activation, nn.Conv2DTranspose)) return nn.Sequential(*decoder) 在这个decoder里加attention,怎么加?
要在decoder里加上attention,可以按照以下步骤进行:
1. 在decoder的输入和输出之间加入attention模块,这可以通过创建一个新的类来实现。例如,你可以创建一个名为`AttentionBlock`的类,该类接受来自encoder的特征图和decoder的上一层输出作为输入,并输出加权后的特征图。
2. 在`create_decoder_block`函数中,将`AttentionBlock`添加到decoder中。具体来说,你可以在每个decoder块的输入和输出之间添加一个`AttentionBlock`。例如,你可以在以下代码段中插入`AttentionBlock`:
```
if i == 0:
# Add attention module here
decoder.append(AttentionBlock(encoder_channels, in_channels))
_in = in_channels * 2
```
3. 在`AttentionBlock`中实现attention逻辑。在这里,你可以使用`nn.Conv2d`和`nn.Linear`层来计算注意力分数,并使用softmax函数将它们归一化到[0, 1]的范围内。然后,你可以将这些分数乘以encoder的特征图,得到加权后的特征图,并将其与decoder的上一层输出相加。
以下是一个示例`AttentionBlock`的代码:
```
class AttentionBlock(nn.Module):
def __init__(self, encoder_channels, decoder_channels):
super(AttentionBlock, self).__init__()
self.conv = nn.Conv2d(encoder_channels + decoder_channels, decoder_channels, kernel_size=1)
self.linear = nn.Linear(decoder_channels, 1)
def forward(self, encoder_features, decoder_features):
# Compute attention scores
batch_size, _, height, width = decoder_features.size()
encoder_features = F.interpolate(encoder_features, size=(height, width))
attention_scores = self.conv(torch.cat([encoder_features, decoder_features], dim=1))
attention_scores = attention_scores.view(batch_size, -1)
attention_scores = self.linear(attention_scores)
attention_scores = attention_scores.view(batch_size, 1, height, width)
attention_scores = F.softmax(attention_scores, dim=-1)
# Apply attention to encoder features
weighted_encoder_features = encoder_features * attention_scores
weighted_encoder_features = weighted_encoder_features.sum(dim=-1).sum(dim=-1).unsqueeze(2).unsqueeze(3)
# Combine with decoder features
combined_features = torch.cat([weighted_encoder_features, decoder_features], dim=1)
return combined_features
```
在这个示例中,我们首先将encoder的特征图插值为与decoder的特征图相同的大小,然后将它们拼接在一起,并通过一个卷积层计算注意力分数。接着,我们将分数归一化,并将它们乘以encoder的特征图,得到加权的特征图。最后,我们将加权的特征图与decoder的上一层输出拼接在一起,并返回结果。
class TemporalModel(nn.Module): def __init__( self, in_channels, receptive_field, input_shape, start_out_channels=64, extra_in_channels=0, n_spatial_layers_between_temporal_layers=0, use_pyramid_pooling=True): super().__init__() self.receptive_field = receptive_field n_temporal_layers = receptive_field - 1 h, w = input_shape modules = [] block_in_channels = in_channels block_out_channels = start_out_channels for _ in range(n_temporal_layers): if use_pyramid_pooling: use_pyramid_pooling = True pool_sizes = [(2, h, w)] else: use_pyramid_pooling = False pool_sizes = None temporal = TemporalBlock( block_in_channels, block_out_channels, use_pyramid_pooling=use_pyramid_pooling, pool_sizes=pool_sizes, ) spatial = [ Bottleneck3D(block_out_channels, block_out_channels, kernel_size=(1, 3, 3)) for _ in range(n_spatial_layers_between_temporal_layers) ] temporal_spatial_layers = nn.Sequential(temporal, *spatial) modules.extend(temporal_spatial_layers) block_in_channels = block_out_channels block_out_channels += extra_in_channels self.out_channels = block_in_channels self.model = nn.Sequential(*modules) def forward(self, x): # Reshape input tensor to (batch, C, time, H, W) x = x.permute(0, 2, 1, 3, 4) x = self.model(x) x = x.permute(0, 2, 1, 3, 4).contiguous() return x[:, (self.receptive_field - 1):]是如何一步步前向传播的?
首先,输入张量x的形状为(batch_size, in_channels, sequence_length, height, width)。
然后,我们将x的维度从(sequence_length, batch_size, in_channels, height, width)改变为(batch_size, sequence_length, in_channels, height, width)。
接下来,我们将模型应用于输入,并将结果从(batch_size, sequence_length, out_channels, height, width)改变为(batch_size, out_channels, sequence_length, height, width),使其与输入张量的形状匹配。
最后,我们将输出张量的维度从(batch_size, out_channels, sequence_length, height, width)改变为(batch_size, sequence_length-receptive_field+1, out_channels, height, width)。其中,sequence_length-receptive_field+1是因为我们使用的是膨胀卷积,其输出序列长度会比输入短receptive_field-1,因此我们需要将其调整为对齐原始输入序列的长度。
阅读全文
相关推荐
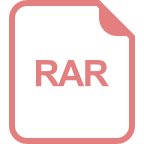














