vue3 ts 字符串操作
时间: 2023-11-09 17:06:20 浏览: 63
在Vue3中使用TypeScript进行字符串操作很简单。以下是一些常见的字符串操作的示例:
1. 字符串拼接:
可以使用模板字符串(template string)或者普通的字符串拼接操作符(+)来实现字符串的拼接。
```typescript
const str1: string = "Hello";
const str2: string = "World";
const result1: string = `${str1} ${str2}`; // 使用模板字符串
const result2: string = str1 + " " + str2; // 使用字符串拼接操作符
```
2. 字符串长度:
可以使用字符串的length属性来获取字符串的长度。
```typescript
const str: string = "Hello";
const length: number = str.length;
console.log(length); // 输出: 5
```
3. 字符串截取:
可以使用slice()方法或者substring()方法来截取字符串的一部分。
```typescript
const str: string = "Hello World";
const substr1: string = str.slice(6, 11); // 使用slice()方法
const substr2: string = str.substring(6, 11); // 使用substring()方法
console.log(substr1); // 输出: World
console.log(substr2); // 输出: World
```
4. 字符串转换大小写:
可以使用toLowerCase()方法将字符串转换为小写,使用toUpperCase()方法将字符串转换为大写。
```typescript
const str: string = "Hello World";const lowerCase: string = str.toLowerCase(); // 转换为小写
const upperCase: string = str.toUpperCase(); // 转换为大写
console.log(lowerCase); // 输出: hello world
console.log(upperCase); // 输出: HELLO WORLD
```
5. 字符串查找:
可以使用indexOf()方法或者includes()方法来查找字符串中是否包含某个子字符串。
```typescript
const str: string = "Hello World";
const index: number = str.indexOf("World"); // 使用indexOf()方法
const includes: boolean = str.includes("World"); // 使用includes()方法
console.log(index); // 输出: 6
console.log(includes); // 输出: true
```
相关推荐
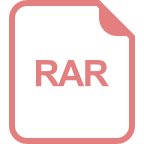
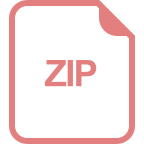
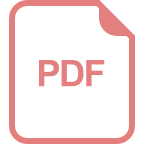














