how to use pyuavcan to simulate
时间: 2024-04-29 09:24:12 浏览: 113
PyUAVCAN is a Python library for working with UAVCAN, a lightweight protocol designed for communication in aerospace and robotics applications. To simulate UAVCAN using PyUAVCAN, follow these steps:
1. Install PyUAVCAN: PyUAVCAN can be installed using pip, the Python package manager. Open a terminal or command prompt and type the following command:
```
pip install pyuavcan
```
2. Create a PyUAVCAN node: A PyUAVCAN node is an instance of the UAVCAN protocol running on a computer. To create a node, import the Node class from the pyuavcan library and create an instance of Node. For example:
```
from pyuavcan.transport import SerialTransport
from pyuavcan.node import Node
transport = SerialTransport("/dev/ttyACM0", baudrate=115200)
node = Node(transport)
```
3. Define a message type: UAVCAN messages are defined in DSDL (Data Structure Description Language) files. You can use the PyUAVCAN DSDL compiler to generate Python classes from DSDL files. For example, if you have a DSDL file called "my_message_type.dsdl", you can generate a Python class by running the following command in a terminal or command prompt:
```
python -m pyuavcan dsdl_compile my_message_type.dsdl
```
This will generate a Python module called "my_message_type" containing the message class.
4. Publish a message: Once you have defined a message type, you can create an instance of the message class and publish it using the PyUAVCAN node. For example, to publish a message of type "my_message_type", you can do the following:
```
from my_message_type import MyMessageType
message = MyMessageType()
message.field1 = 123
message.field2 = "hello"
node.broadcast(message)
```
This will broadcast the message to all nodes on the network.
5. Subscribe to messages: You can also use the PyUAVCAN node to subscribe to messages of a particular type. For example, to subscribe to messages of type "my_message_type", you can do the following:
```
from my_message_type import MyMessageType
def my_callback(event):
message = event.message
print("Received message:", message)
node.add_handler(MyMessageType, my_callback)
```
This will register a callback function that will be called whenever a message of type "my_message_type" is received.
These are the basic steps for simulating UAVCAN using PyUAVCAN. You can customize the simulation by using different transport protocols (such as UDP or CAN) and defining your own message types.
阅读全文
相关推荐
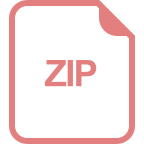
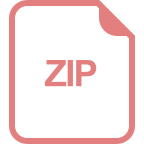
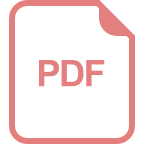
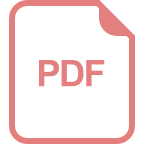
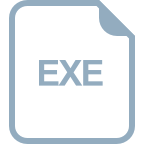
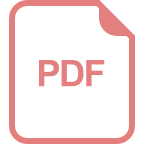
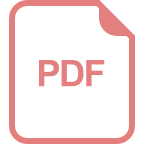
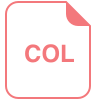






