python计算图片中颜色比例
时间: 2023-10-07 08:09:47 浏览: 141
你可以使用Python的Pillow库来计算图片中每种颜色的比例。以下是一个简单的示例代码:
```python
from PIL import Image
# 打开图片
image = Image.open("image.jpg")
# 将图片转换为RGB模式
image = image.convert("RGB")
# 获取图片中每个像素的颜色值
colors = image.getcolors(image.size[0] * image.size[1])
# 计算每种颜色的比例
total_pixels = image.size[0] * image.size[1]
for color in colors:
percentage = color[0] / total_pixels * 100
print(f"颜色 {color[1]} 占比 {percentage:.2f}%")
```
这段代码打印出每种颜色在图片中的占比。你可以将`"image.jpg"`替换为你想要计算的图片路径。
相关问题
python计算图片中除去黑色外的颜色比例
可以使用Python的Pillow库来读取图片并计算颜色比例。以下是一个简单的示例代码,可以计算除黑色外的颜色比例。
```python
from PIL import Image
# 打开图片
im = Image.open('image.png')
# 获取图片颜色直方图
histogram = im.histogram()
# 计算除黑色外的颜色总数
total_pixels = sum(histogram)
black_pixels = histogram[0]
color_pixels = total_pixels - black_pixels
# 计算颜色比例
color_ratio = color_pixels / total_pixels
print(f"除黑色外的颜色比例为:{color_ratio}")
```
注意,这里假设黑色的像素值为0。如果黑色的像素值不是0,则需要相应地修改代码。另外,这个计算方法并不考虑不同颜色之间的权重,只是简单地计算除黑色外的像素数量占总像素数量的比例。
python计算图片中除去黑色的各种颜色比例
你可以使用Python的Pillow库来计算图片中除去黑色的各种颜色比例。以下是一个示例代码:
```python
from PIL import Image
# 打开图片
image = Image.open("example.jpg")
# 获取图片的大小
width, height = image.size
# 初始化颜色计数器
color_count = {
"red": 0,
"green": 0,
"blue": 0,
"yellow": 0,
"purple": 0,
"white": 0,
"gray": 0
}
# 统计颜色数量
for x in range(width):
for y in range(height):
# 获取像素点的 RGB 值
r, g, b = image.getpixel((x, y))
# 排除黑色像素
if r + g + b > 0:
# 计算颜色比例
total = r + g + b
red_ratio = r / total
green_ratio = g / total
blue_ratio = b / total
# 判断颜色类型并增加计数器
if red_ratio > 0.8 and green_ratio < 0.2 and blue_ratio < 0.2:
color_count["red"] += 1
elif red_ratio < 0.2 and green_ratio > 0.8 and blue_ratio < 0.2:
color_count["green"] += 1
elif red_ratio < 0.2 and green_ratio < 0.2 and blue_ratio > 0.8:
color_count["blue"] += 1
elif red_ratio > 0.8 and green_ratio > 0.8 and blue_ratio < 0.2:
color_count["yellow"] += 1
elif red_ratio > 0.8 and green_ratio < 0.2 and blue_ratio > 0.8:
color_count["purple"] += 1
elif red_ratio > 0.8 and green_ratio > 0.8 and blue_ratio > 0.8:
color_count["white"] += 1
else:
color_count["gray"] += 1
# 计算颜色比例
total_count = sum(color_count.values())
color_ratio = {k: v / total_count for k, v in color_count.items()}
print(color_ratio)
```
这段代码会统计图片中红色、绿色、蓝色、黄色、紫色、白色和灰色像素的数量,并计算它们的比例,最后输出一个字典,其中键是颜色名称,值是颜色比例。你可以根据需要修改代码,例如添加更多的颜色类型或修改颜色比例的计算方式。
相关推荐
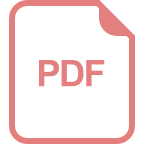
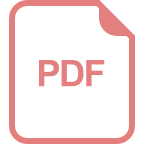
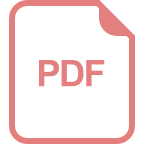











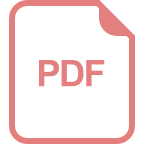