java中的FileDialog类的作用和示例
时间: 2024-03-28 10:41:59 浏览: 60
Java中的FileDialog类是用于创建文件对话框的类,它可以让用户选择一个或多个文件,或者指定一个文件保存路径。该类继承自Dialog类,因此可以使用Dialog类的所有方法和属性。
以下是一个使用FileDialog类的示例:
```java
import java.awt.*;
import java.awt.event.*;
import java.io.*;
public class FileDialogExample extends Frame implements ActionListener {
private TextArea textArea;
private Button openButton;
private Button saveButton;
public FileDialogExample() {
super("FileDialog Example");
textArea = new TextArea();
add(textArea, BorderLayout.CENTER);
openButton = new Button("Open");
openButton.addActionListener(this);
add(openButton, BorderLayout.WEST);
saveButton = new Button("Save");
saveButton.addActionListener(this);
add(saveButton, BorderLayout.EAST);
setSize(400, 300);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == openButton) {
FileDialog dialog = new FileDialog(this, "Open File", FileDialog.LOAD);
dialog.setVisible(true);
String filePath = dialog.getDirectory() + dialog.getFile();
File file = new File(filePath);
try {
BufferedReader reader = new BufferedReader(new FileReader(file));
String line;
while ((line = reader.readLine()) != null) {
textArea.append(line + "\n");
}
reader.close();
} catch (IOException ex) {
ex.printStackTrace();
}
} else if (e.getSource() == saveButton) {
FileDialog dialog = new FileDialog(this, "Save File", FileDialog.SAVE);
dialog.setVisible(true);
String filePath = dialog.getDirectory() + dialog.getFile();
File file = new File(filePath);
try {
BufferedWriter writer = new BufferedWriter(new FileWriter(file));
writer.write(textArea.getText());
writer.close();
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
public static void main(String[] args) {
new FileDialogExample();
}
}
```
在这个示例中,我们创建了一个带有文本区域、打开按钮和保存按钮的窗口。当用户点击打开按钮时,我们创建一个FileDialog对象,设置对话框的标题为“Open File”,对话框的模式为LOAD(表示用户可以选择一个或多个文件),并显示对话框。用户选择文件后,我们读取文件的内容,并将其显示在文本区域中。
当用户点击保存按钮时,我们创建一个FileDialog对象,设置对话框的标题为“Save File”,对话框的模式为SAVE(表示用户可以指定一个保存路径),并显示对话框。用户指定保存路径后,我们将文本区域中的内容写入一个文件中。
通过这个示例,我们可以看到FileDialog类的基本用法,它可以方便地让用户选择文件,或者指定文件的保存路径。
阅读全文
相关推荐
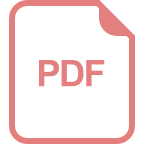
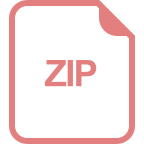

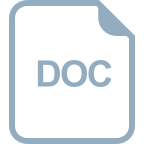
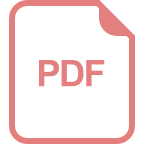
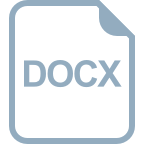
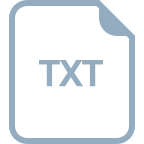
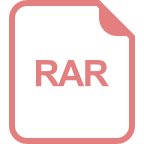
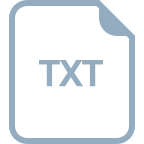
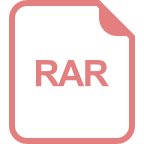
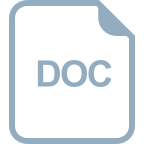
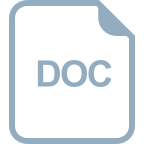
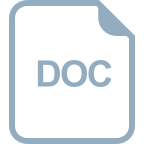
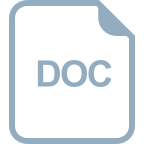
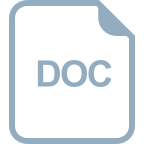


