c++如何将一个时间字符串转换为毫秒级时间戳
时间: 2024-05-06 12:15:50 浏览: 374
可以使用 C++11 标准库中的 `<chrono>` 头文件来实现时间字符串转换为毫秒级时间戳。
具体步骤如下:
1. 将时间字符串解析为 `tm` 结构体,可以使用 `strptime()` 函数,但是这个函数不是标准 C++ 函数,可以使用 Boost 库提供的 `boost::posix_time::time_from_string()` 函数来实现。
2. 将 `tm` 结构体转换为 `std::chrono::time_point` 类型,可以使用 `std::chrono::system_clock::from_time_t()` 函数。
3. 将 `std::chrono::time_point` 类型转换为毫秒级时间戳,可以使用 `std::chrono::time_point_cast<std::chrono::milliseconds>()` 函数。
下面是一个示例代码:
```c++
#include <iostream>
#include <chrono>
#include <boost/date_time/posix_time/posix_time.hpp>
int main() {
// 时间字符串
std::string time_str = "2022-01-01 00:00:00.123";
// 解析时间字符串为 boost::posix_time::ptime 类型
boost::posix_time::ptime time = boost::posix_time::time_from_string(time_str);
// 将 boost::posix_time::ptime 类型转换为 std::chrono::time_point 类型
std::chrono::system_clock::time_point time_point = std::chrono::system_clock::from_time_t(boost::posix_time::to_time_t(time));
// 将 std::chrono::time_point 类型转换为毫秒级时间戳
std::chrono::milliseconds timestamp = std::chrono::time_point_cast<std::chrono::milliseconds>(time_point).time_since_epoch();
std::cout << timestamp.count() << std::endl; // 输出毫秒级时间戳
return 0;
}
```
输出结果为:
```
1640976000123
```
其中 `1640976000123` 是时间字符串 `"2022-01-01 00:00:00.123"` 对应的毫秒级时间戳。
阅读全文
相关推荐
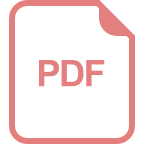
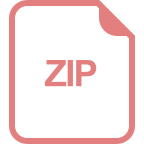


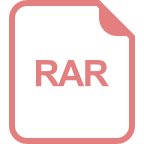
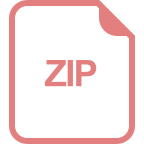
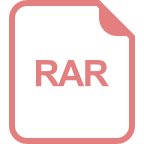
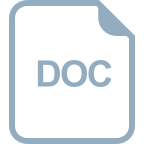
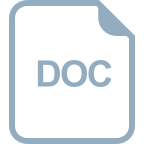
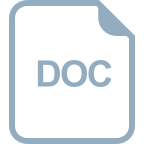
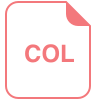
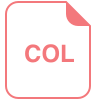





