用python实现亚像素边缘检测
时间: 2023-10-14 20:05:46 浏览: 96
可以使用OpenCV库中的cv2.Sobel()函数进行亚像素边缘检测,具体实现方法如下:
```python
import cv2
# 读取图像并转为灰度图像
img = cv2.imread('image.jpg')
gray = cv2.cvtColor(img,cv2.COLOR_BGR2GRAY)
# 使用Sobel算子进行亚像素边缘检测
x = cv2.Sobel(gray,cv2.CV_16S,1,0)
y = cv2.Sobel(gray,cv2.CV_16S,0,1)
absx = cv2.convertScaleAbs(x)
absy = cv2.convertScaleAbs(y)
result = cv2.addWeighted(absx,0.5,absy,0.5,0)
# 显示结果
cv2.imshow('result',result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
希望可以帮到你!接下来还有什么问题吗?
相关问题
亚像素边缘检测python
亚像素边缘检测是一种边缘检测算法,它可以在像素级别上检测图像中的边缘。Python中有许多库和工具可用于实现亚像素边缘检测,下面是一个使用OpenCV库实现亚像素边缘检测的示例代码:
``` python
import cv2
# 读取图像
img = cv2.imread('image.jpg')
# 转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 计算梯度
sobelx = cv2.Sobel(gray, cv2.CV_64F, 1, 0, ksize=3)
sobely = cv2.Sobel(gray, cv2.CV_64F, 0, 1, ksize=3)
# 计算梯度的大小和方向
mag, angle = cv2.cartToPolar(sobelx, sobely, angleInDegrees=True)
# 应用非极大值抑制
kernel_size = 3
m, n = mag.shape
for i in range(1, m - 1):
for j in range(1, n - 1):
if (angle[i, j] >= 0 and angle[i, j] < 22.5) or (angle[i, j] >= 157.5 and angle[i, j] <= 180):
a = mag[i, j - 1]
b = mag[i, j]
c = mag[i, j + 1]
elif (angle[i, j] >= 22.5 and angle[i, j] < 67.5):
a = mag[i - 1, j - 1]
b = mag[i, j]
c = mag[i + 1, j + 1]
elif (angle[i, j] >= 67.5 and angle[i, j] < 112.5):
a = mag[i - 1, j]
b = mag[i, j]
c = mag[i + 1, j]
elif (angle[i, j] >= 112.5 and angle[i, j] < 157.5):
a = mag[i - 1, j + 1]
b = mag[i, j]
c = mag[i + 1, j - 1]
if (b > a and b > c):
mag[i, j] = b
else:
mag[i, j] = 0
# 应用双阈值算法
low_threshold = 0.05 * mag.max()
high_threshold = 0.15 * mag.max()
threshold = cv2.Canny(img, low_threshold, high_threshold)
# 应用亚像素边缘检测
subpixel_threshold = 0.5
lines = cv2.HoughLinesP(threshold, 1, cv2.PI / 180, 50, minLineLength=50, maxLineGap=5)
for line in lines:
x1, y1, x2, y2 = line[0]
dx = x2 - x1
dy = y2 - y1
length = cv2.sqrt(dx * dx + dy * dy)[0]
if length > 0:
vx = dx / length
vy = dy / length
x = x1
y = y1
while length > 0:
length -= subpixel_threshold
x += subpixel_threshold * vx
y += subpixel_threshold * vy
cv2.circle(img, (int(x), int(y)), 1, (0, 255, 0), -1)
# 显示结果
cv2.imshow('result', img)
cv2.waitKey(0)
```
这段代码首先读取图像并将其转换为灰度图像,然后计算图像的梯度,应用非极大值抑制和双阈值算法来检测边缘,最后使用Hough变换和亚像素边缘检测来检测和绘制边缘。
插值法亚像素边缘检测python
可以使用插值法进行亚像素边缘检测的Python库是OpenCV。OpenCV提供了一个函数cv2.Canny(),它可以使用插值法来检测图像中的边缘。
下面是一个示例代码,展示了如何使用插值法进行亚像素边缘检测:
```python
import cv2
# 读取图像
image = cv2.imread('image.jpg', 0)
# 对图像进行高斯模糊
blurred = cv2.GaussianBlur(image, (5, 5), 0)
# 使用Canny函数进行边缘检测
edges = cv2.Canny(blurred, 30, 70)
# 显示结果
cv2.imshow('Original Image', image)
cv2.imshow('Edges', edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这个示例中,首先读取了一张灰度图像。然后对图像进行了高斯模糊,以降低噪声的影响。最后使用cv2.Canny()函数进行边缘检测,将检测到的边缘显示出来。
相关推荐
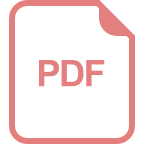
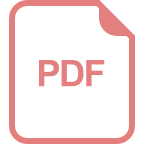












