亚像素边缘检测opencv
时间: 2023-08-30 14:11:54 浏览: 158
亚像素边缘检测是一种改进的边缘检测算法,可以在像素级别更准确地定位边缘。在OpenCV中,可以使用以下步骤进行亚像素边缘检测:
1. 读取图像并转换为灰度图像。
2. 对图像进行高斯滤波以减少噪声。
3. 使用Sobel算子进行水平和垂直方向的边缘检测。
4. 对Sobel结果进行非极大值抑制,去除非边缘像素。
5. 使用Hough变换检测直线。
6. 使用亚像素精确化技术对边缘进行细化。
以下是一个示例代码,展示如何在OpenCV中实现亚像素边缘检测:
```python
import cv2
import numpy as np
def subpixel_edge_detection(image):
# Convert to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Apply Gaussian blur
blurred = cv2.GaussianBlur(gray, (3, 3), 0)
# Perform Sobel edge detection
sobelx = cv2.Sobel(blurred, cv2.CV_64F, 1, 0, ksize=3)
sobely = cv2.Sobel(blurred, cv2.CV_64F, 0, 1, ksize=3)
# Calculate gradient magnitude and direction
grad_mag = np.sqrt(sobelx**2 + sobely**2)
grad_dir = np.arctan2(sobely, sobelx)
# Non-maximum suppression
grad_max = cv2.morphologyEx(grad_mag, cv2.MORPH_CLOSE, np.ones((3,3)))
non_max = cv2.compare(grad_max, grad_mag, cv2.CMP_EQ)
grad_suppressed = grad_mag * non_max
# Hough line detection
lines = cv2.HoughLinesP(grad_suppressed.astype('uint8'), 1, np.pi/180, threshold=50, minLineLength=50, maxLineGap=10)
# Subpixel refinement
for line in lines:
x1, y1, x2, y2 = line[0]
cv2.line(image, (x1, y1), (x2, y2), (0, 255, 0), 2)
return image
# Read image
image = cv2.imread('image.jpg')
# Perform subpixel edge detection
result = subpixel_edge_detection(image)
# Display result
cv2.imshow('Subpixel Edge Detection', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
请注意,此示例代码仅为演示目的,实际应用中可能需要针对特定场景进行参数调整和优化。
阅读全文
相关推荐
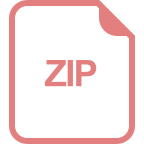
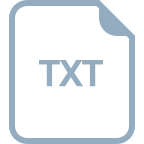
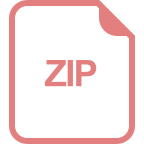















