用matlab实现dijsktra 算法
时间: 2023-07-12 07:25:22 浏览: 59
以下是用 Matlab 实现 Dijkstra 算法的示例代码:
```matlab
function [dist, path] = dijkstra(adj, src, dest)
% DIJKSTRA Find the shortest path from a source node to a destination node
% in a graph represented by an adjacency matrix using Dijkstra's algorithm.
% adj: adjacency matrix of the graph
% src: source node index
% dest: destination node index
% dist: shortest distance from the source node to each node
% path: shortest path from the source node to the destination node
%
% Example:
% adj = [0 3 9 0 0;
% 3 0 1 7 0;
% 9 1 0 5 8;
% 0 7 5 0 4;
% 0 0 8 4 0];
% [dist, path] = dijkstra(adj, 1, 5);
n = size(adj, 1); % number of nodes
dist = inf(1, n); % initialize distances to infinity
prev = zeros(1, n); % initialize previous node indices to zero
visited = false(1, n); % initialize visited flags to false
dist(src) = 0; % distance from source to itself is zero
for i = 1:n
% find the node with the smallest tentative distance
[min_dist, u] = min(dist(~visited));
if isempty(u)
break; % all remaining nodes are unreachable
end
% mark the selected node as visited
visited(u) = true;
% update the distances of its neighbors
for v = 1:n
if adj(u, v) ~= 0 && ~visited(v)
alt = dist(u) + adj(u, v);
if alt < dist(v)
dist(v) = alt;
prev(v) = u;
end
end
end
% check if we have reached the destination node
if u == dest
break;
end
end
% construct the shortest path from the source to the destination
path = [];
u = dest;
while prev(u) ~= 0
path = [u path];
u = prev(u);
end
if u == src
path = [u path];
end
end
```
该函数的输入参数是邻接矩阵 `adj`,源节点索引 `src` 和目标节点索引 `dest`,输出参数是最短距离数组 `dist` 和最短路径数组 `path`。该函数使用了 Dijkstra 算法来计算最短路径,其中包括了对节点距离的初始化、标记已访问的节点、更新邻居节点的距离等过程。最后,根据存储的前驱节点信息,构造从源节点到目标节点的最短路径。
相关推荐
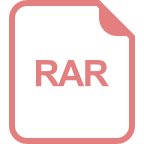
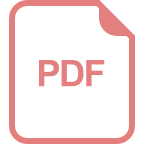














